Creating a Lightbox with JavaScript
Published June 23, 2024 at 8:22 pm
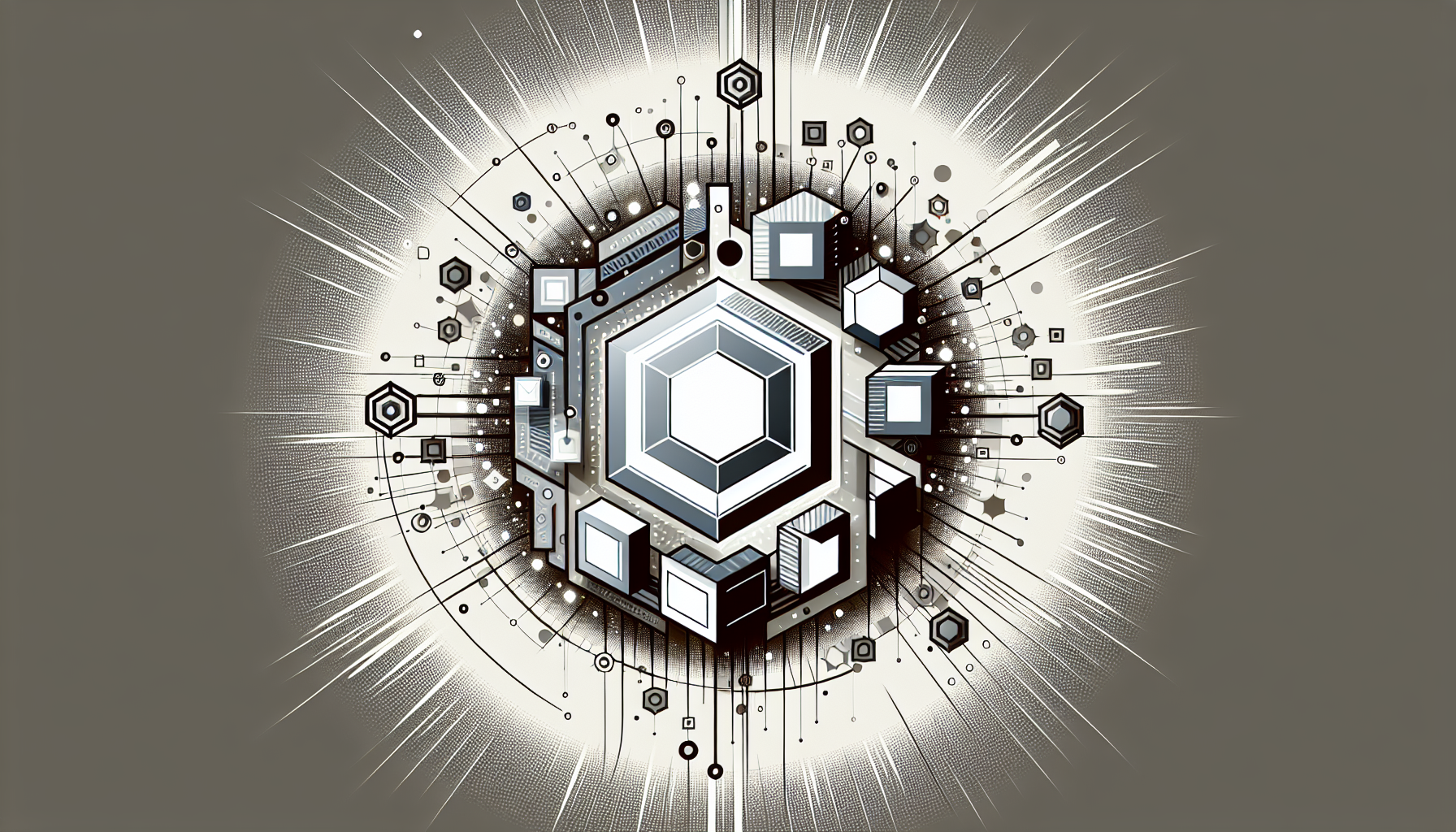
What is a Lightbox?
A lightbox is a way to display images and videos on the web by overlaying them on the current page.
It is often used in photo galleries, portfolios, and e-commerce sites.
The lightbox effect enhances the user experience by providing a focused view of the content.
Users can click on a thumbnail to see a larger view without navigating away from the current page.
How to Create a Lightbox with JavaScript
Creating a lightbox with JavaScript involves using HTML, CSS, and JavaScript to build the structure and functionality.
This step-by-step guide will walk you through how to create a basic lightbox.
TLDR: Quick Code to Implement a Lightbox
// HTML Part



/* CSS Part */
/* styles.css */
body {
font-family: Arial, sans-serif;
}
.gallery {
display: flex;
justify-content: center;
margin-top: 50px;
}
.thumbnail {
width: 100px;
margin: 0 10px;
cursor: pointer;
}
.lightbox {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.8);
justify-content: center;
align-items: center;
}
.lightbox img.lightbox-content {
max-width: 90%;
max-height: 90%;
}
.lightbox .close {
position: absolute;
top: 20px;
right: 20px;
color: white;
font-size: 30px;
cursor: pointer;
}
/* JavaScript Part */
/* script.js */
document.addEventListener('DOMContentLoaded', function() {
const gallery = document.querySelector('.gallery');
const lightbox = document.getElementById('lightbox');
const lightboxContent = document.querySelector('.lightbox-content');
const closeBtn = document.querySelector('.close');
gallery.addEventListener('click', function(e) {
if (e.target.classList.contains('thumbnail')) {
lightbox.style.display = 'flex';
lightboxContent.src = e.target.src;
}
});
closeBtn.addEventListener('click', function() {
lightbox.style.display = 'none';
});
lightbox.addEventListener('click', function(e) {
if (e.target === lightbox) {
lightbox.style.display = 'none';
}
});
});
Step-by-Step Guide to Building a Lightbox
We will break down the process into manageable steps.
Step 1: Set up the HTML Structure
Create the basic HTML structure in your HTML file.
Add the following code inside the <body>
tag:



Step 2: Style the Lightbox with CSS
Use the following CSS to style the lightbox.
Add this to your CSS file to give it the desired look and feel:
body {
font-family: Arial, sans-serif;
}
.gallery {
display: flex;
justify-content: center;
margin-top: 50px;
}
.thumbnail {
width: 100px;
margin: 0 10px;
cursor: pointer;
}
.lightbox {
display: none;
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.8);
justify-content: center;
align-items: center;
}
.lightbox img.lightbox-content {
max-width: 90%;
max-height: 90%;
}
.lightbox .close {
position: absolute;
top: 20px;
right: 20px;
color: white;
font-size: 30px;
cursor: pointer;
}
Step 3: Add Functionality with JavaScript
Implement the functionality using JavaScript.
Add this code to your JavaScript file:
document.addEventListener('DOMContentLoaded', function() {
const gallery = document.querySelector('.gallery');
const lightbox = document.getElementById('lightbox');
const lightboxContent = document.querySelector('.lightbox-content');
const closeBtn = document.querySelector('.close');
gallery.addEventListener('click', function(e) {
if (e.target.classList.contains('thumbnail')) {
lightbox.style.display = 'flex';
lightboxContent.src = e.target.src;
}
});
closeBtn.addEventListener('click', function() {
lightbox.style.display = 'none';
});
lightbox.addEventListener('click', function(e) {
if (e.target === lightbox) {
lightbox.style.display = 'none';
}
});
});
How Does the JavaScript Work?
The JavaScript code listens for a click event on the gallery container.
When a thumbnail image is clicked, it displays the lightbox with the clicked image.
The close button and clicking outside the image will close the lightbox.
Tips & Tricks for Improving Your Lightbox
Add transitions to enhance visual appeal:
.lightbox img.lightbox-content {
transition: transform 0.3s ease-in-out;
}
.lightbox.open img.lightbox-content {
transform: scale(1.1);
}
Consider lazy loading your images:
This will speed up the initial load time of your page.
Common Issues and Troubleshooting
**Issue:** Lightbox doesn’t open.
**Solution:** Ensure you have linked your JavaScript and CSS files correctly.
**Issue:** Images are not centered.
**Solution:** Check the alignment styles in your CSS file.
FAQs
How can I add captions to my lightbox images?
Add a caption element to your lightbox and set its content based on the clicked image.
Is it possible to navigate between images within the lightbox?
Yes, you can add navigation buttons and implement scrolling functionality in JavaScript.
Can I use this lightbox with videos?
Yes, you can adapt the code to display videos by changing the lightbox content type.
How do I make the lightbox responsive?
Use CSS media queries to adjust the lightbox design for different screen sizes.
Adding Animations and Enhancements
Animations can enhance the user experience, making your lightbox more engaging.
Let’s add some smooth transitions to the lightbox to make it more visually appealing.
Add the following CSS to your stylesheet:
.lightbox {
transition: opacity 0.3s ease;
}
.lightbox.open {
opacity: 1;
}
.lightbox img.lightbox-content {
transition: transform 0.3s ease-in-out;
}
.lightbox.open img.lightbox-content {
transform: scale(1);
}
Update your JavaScript to toggle the open
class:
document.addEventListener('DOMContentLoaded', function() {
const gallery = document.querySelector('.gallery');
const lightbox = document.getElementById('lightbox');
const lightboxContent = document.querySelector('.lightbox-content');
const closeBtn = document.querySelector('.close');
gallery.addEventListener('click', function(e) {
if (e.target.classList.contains('thumbnail')) {
lightbox.style.display = 'flex';
setTimeout(() => lightbox.classList.add('open'), 10); // Delay to trigger the transition
lightboxContent.src = e.target.src;
}
});
closeBtn.addEventListener('click', function() {
lightbox.classList.remove('open');
setTimeout(() => lightbox.style.display = 'none', 300); // Match the transition time
});
lightbox.addEventListener('click', function(e) {
if (e.target === lightbox) {
lightbox.classList.remove('open');
setTimeout(() => lightbox.style.display = 'none', 300); // Match the transition time
}
});
});
Making the Lightbox Responsive
To ensure your lightbox looks great on all devices, you should use CSS media queries.
Here are some responsive design tips:
Add the following media queries to your CSS:
@media (max-width: 600px) {
.gallery {
flex-direction: column;
}
.thumbnail {
width: 90%;
margin: 10px 0;
}
.lightbox .close {
font-size: 20px;
top: 10px;
right: 10px;
}
}
These styles will ensure your gallery and lightbox adapt to smaller screens.
Adjust the dimensions and other properties as needed.
Handling Image Loading
Large images can take time to load, causing delays in displaying the lightbox.
You can implement lazy loading to improve performance.
Here’s how to do it:
Add a data-full
attribute to your thumbnail images:
Update your JavaScript to use the full image source:
document.addEventListener('DOMContentLoaded', function() {
const gallery = document.querySelector('.gallery');
const lightbox = document.getElementById('lightbox');
const lightboxContent = document.querySelector('.lightbox-content');
const closeBtn = document.querySelector('.close');
gallery.addEventListener('click', function(e) {
if (e.target.classList.contains('thumbnail')) {
lightbox.style.display = 'flex';
setTimeout(() => lightbox.classList.add('open'), 10); // Delay to trigger the transition
lightboxContent.src = e.target.getAttribute('data-full');
}
});
closeBtn.addEventListener('click', function() {
lightbox.classList.remove('open');
setTimeout(() => lightbox.style.display = 'none', 300); // Match the transition time
});
lightbox.addEventListener('click', function(e) {
if (e.target === lightbox) {
lightbox.classList.remove('open');
setTimeout(() => lightbox.style.display = 'none', 300); // Match the transition time
}
});
});
FAQs Section
How can I customize the lightbox close button?
You can style the close button using CSS by targeting the .close
class.
Is there a way to add carousel functionality to the lightbox?
Yes, you can add navigation buttons and use JavaScript to change the lightbox content.
How can I preload images for a smoother experience?
Use JavaScript to preload images in the background before the user clicks on the thumbnails.
Can I use this lightbox setup for video content?
Yes, modify the lightbox-content
to be a video element and update JavaScript accordingly.
What if I want to show captions with the images?
Add a caption element inside the lightbox and use JavaScript to set the caption text when an image is clicked.
Shop more on Amazon