Building a Custom Context Menu with JavaScript
Published June 30, 2024 at 4:36 pm
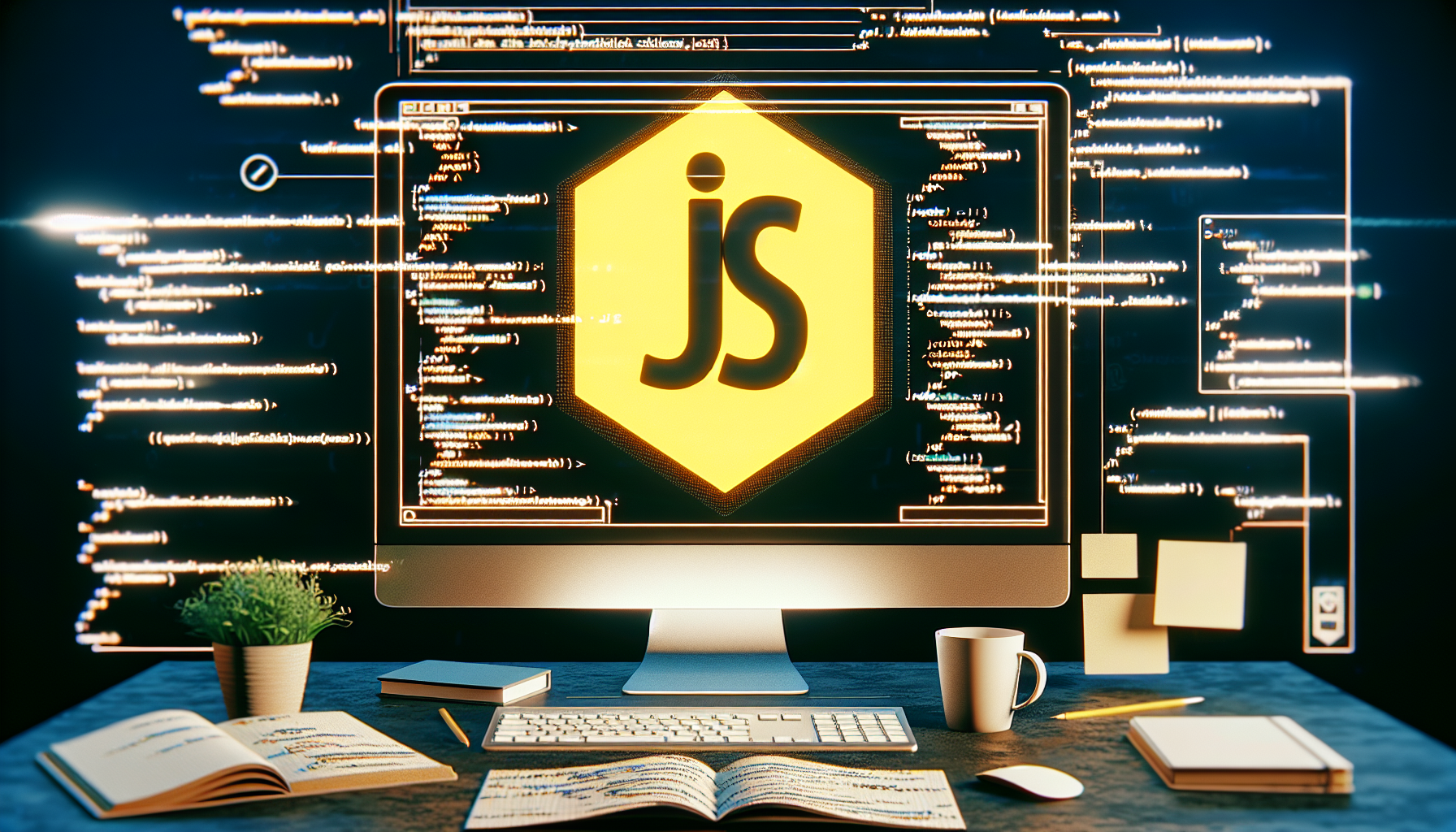
Introduction to Building a Custom Context Menu with JavaScript
Are you tired of the default context menu in your web application and wondering how to create a custom one? Custom context menus offer a unique user experience by adding custom features specific to your needs.
This guide will show you how to build a custom context menu using JavaScript, which can be tailored to fit various requirements.
TL;DR: How to Create a Custom Context Menu in JavaScript?
Here’s a quick example of a basic custom context menu:
// Create the context menu HTML
var menu = document.createElement('div');
menu.id = 'customContextMenu';
menu.innerHTML = '
- Option 1
- Option 2
';
document.body.appendChild(menu);
// Style the menu via CSS
var style = document.createElement('style');
style.innerHTML = '#customContextMenu { position: absolute; background: #fff; border: 1px solid #ccc; display: none; z-index: 1000; } #customContextMenu ul { list-style: none; margin: 0; padding: 5px; } #customContextMenu li { padding: 8px 12px; cursor: pointer; }';
document.head.appendChild(style);
// Add event listeners to display menu
document.addEventListener('contextmenu', function(event) {
event.preventDefault();
menu.style.top = event.pageY + 'px';
menu.style.left = event.pageX + 'px';
menu.style.display = 'block';
});
// Hide the menu on click outside
document.addEventListener('click', function() {
menu.style.display = 'none';
});
This example creates a simple custom context menu with two options that display when you right-click on the page.
What is a Context Menu?
A context menu, often called a right-click menu, provides quick access to a set of actions related to an element on which the user right-clicks.
In web development, the default context menus are provided by the browser, which might not always align with your application’s needs.
By customizing the context menu, you can provide specific actions that improve the user experience.
Building Blocks for Custom Context Menus
To create custom context menus, you’ll need to understand a few key concepts such as DOM manipulation, event listeners, and CSS positioning.
Let’s break down these concepts to build a simple yet effective context menu.
Creating the Menu Structure
You start by creating the HTML structure for the context menu. This typically will be a <div>
element containing a list of actions.
var menu = document.createElement('div');
menu.id = 'customContextMenu';
menu.innerHTML = '
- Option 1
- Option 2
';
document.body.appendChild(menu);
Here, a <div>
element with the ID 'customContextMenu'
is created and appended to the body. The inner HTML is set to an unordered list with two options.
Styling the Context Menu
It’s essential to style your context menu so that it appears where and how you want it.
You can use CSS for positioning, background colors, borders, and other styles:
var style = document.createElement('style');
style.innerHTML = '#customContextMenu { position: absolute; background: #fff; border: 1px solid #ccc; display: none; z-index: 1000; } #customContextMenu ul { list-style: none; margin: 0; padding: 5px; } #customContextMenu li { padding: 8px 12px; cursor: pointer; }';
document.head.appendChild(style);
This CSS ensures that the menu is positioned absolutely, is initially hidden, and has a white background with a border.
Displaying the Menu on Right-Click
The primary functionality of a context menu is to display it when the user right-clicks.
You can achieve this using the contextmenu
event in JavaScript:
document.addEventListener('contextmenu', function(event) {
event.preventDefault();
menu.style.top = event.pageY + 'px';
menu.style.left = event.pageX + 'px';
menu.style.display = 'block';
});
Here, event.preventDefault()
stops the default browser menu from opening, and the custom menu’s position is set based on the mouse coordinates.
Hiding the Menu
To ensure a smooth user experience, the menu should disappear when the user clicks anywhere outside of it.
This can be implemented with another event listener on the click
event:
document.addEventListener('click', function() {
menu.style.display = 'none';
});
This ensures that the custom context menu hides whenever the user clicks elsewhere on the page.
Enhancing the Custom Context Menu
Once the basic functionality is in place, you can enhance the context menu with additional features like submenus, keyboard shortcuts, or item-specific actions.
For example, adding event listeners to the menu items can trigger specific actions:
menu.querySelectorAll('li').forEach(function(item) {
item.addEventListener('click', function() {
alert('You clicked on ' + this.textContent);
menu.style.display = 'none';
});
});
This snippet adds a click event listener to each menu item, displaying an alert with the item’s text when clicked and hiding the menu.
Context Menu for Specific Elements
It’s often more user-friendly to restrict the context menu to specific elements rather than the entire page.
Instead of the document, attach the event listener to a specific element:
var specificElement = document.getElementById('specificElement');
specificElement.addEventListener('contextmenu', function(event) {
event.preventDefault();
menu.style.top = event.pageY + 'px';
menu.style.left = event.pageX + 'px';
menu.style.display = 'block';
});
document.addEventListener('click', function() {
menu.style.display = 'none';
});
Here, right-clicking on specificElement
will display the custom context menu, and the menu will still hide when clicking outside.
Pros and Cons of Custom Context Menus
Advantages:
- Custom context menus provide a tailored user experience, allowing for application-specific actions.
- They can be styled and positioned to better integrate with your application’s design.
Drawbacks:
- Developing and maintaining custom context menus can be more time-consuming than using default browser menus.
- They may not be as accessible if not implemented correctly, requiring additional consideration for accessibility standards.
FAQs Related to Custom Context Menus in JavaScript
How can I make the context menu accessible?
Ensure your context menu is keyboard-navigable by using tabindex and ARIA roles.
Can I add dynamic content to the context menu?
Yes, you can use JavaScript to dynamically update the content of the menu before displaying it.
How do I handle multiple context menus on the same page?
Create separate context menu elements and manage their visibility using similar event listener techniques.
Can I use CSS frameworks to style my context menu?
Yes, CSS frameworks like Bootstrap can be used to enhance the visual appearance of your custom context menu.
Is it possible to disable the default context menu in specific parts of my application?
Yes, you can use the contextmenu
event and event.preventDefault()
to disable the default menu in specified areas.
Enhancing User Experience with Custom Context Menus
No application is complete without providing some level of custom functionality that matches the specific needs of its users.
Custom context menus can be an engaging way to provide quick access to relevant functions.
With custom context menus, users are presented with options that are specific to the context, making their interaction more efficient and intuitive.
Advanced Context Menu Features
Now that we have a basic custom context menu, let’s look at some advanced features you can add.
One of the most common enhancements is adding submenus to context menu options.
Implementing Submenus
To add submenus, you’ll need to nest additional <ul>
elements within the context menu’s <li>
elements.
For example:
// Create the context menu with submenus
var menu = document.createElement('div');
menu.id = 'customContextMenu';
menu.innerHTML = `
- Option 1
- Option 2
- Sub Option 1
- Sub Option 2
`;
document.body.appendChild(menu);
var style = document.createElement('style');
style.innerHTML = `
#customContextMenu { position: absolute; background: #fff; border: 1px solid #ccc; display: none; z-index: 1000; }
#customContextMenu ul { list-style: none; margin: 0; padding: 5px; }
#customContextMenu li { padding: 8px 12px; cursor: pointer; position: relative; }
#customContextMenu li ul { position: absolute; top: 0; left: 100%; background: #fff; border: 1px solid #ccc; display: none; }
#customContextMenu li:hover ul { display: block; }
`;
document.head.appendChild(style);
In this example, hovering over “Option 2” reveals a nested submenu with “Sub Option 1” and “Sub Option 2”.
Keyboard Accessibility
Accessibility is crucial for usability.
You can make the custom context menu accessible via keyboard navigation by using tabindex
and ARIA attributes:
// Enhancing accessibility with tabindex and ARIA roles
menu.querySelectorAll('li').forEach(function(item) {
item.setAttribute('tabindex', '0');
item.setAttribute('role', 'menuitem');
item.addEventListener('keydown', function(event) {
if (event.key === 'Enter') {
alert('You selected ' + this.textContent);
menu.style.display = 'none';
}
});
});
This snippet sets up the context menu items to be focusable and adds keyboard support for the Enter key.
Dynamic Context Menu Content
Sometimes, you’ll need the context menu content to be dynamic, reflecting the state or data of your application.
You can achieve this by updating the menu content before displaying it:
// Example: Creating dynamic menu content
document.addEventListener('contextmenu', function(event) {
event.preventDefault();
var dynamicContent = `
- Dynamic Option 1
- Dynamic Option 2
`;
menu.innerHTML = dynamicContent;
menu.style.top = event.pageY + 'px';
menu.style.left = event.pageX + 'px';
menu.style.display = 'block';
});
This code dynamically updates the custom context menu content before it’s shown.
Context Menus with Conditional Logic
If your application requires context menus to change based on the specific element being right-clicked, use conditional logic.
Attach event listeners to elements and update the menu accordingly:
// Example: Context menu with conditional logic
document.addEventListener('contextmenu', function(event) {
event.preventDefault();
if (event.target.classList.contains('special-element')) {
menu.innerHTML = `
- Special Option 1
- Special Option 2
`;
} else {
menu.innerHTML = `
- Default Option 1
- Default Option 2
`;
}
menu.style.top = event.pageY + 'px';
menu.style.left = event.pageX + 'px';
menu.style.display = 'block';
});
This example updates the context menu according to whether a special element was right-clicked.
Debugging Common Issues with Custom Context Menus
Custom context menus can have bugs and issues. Here are some common problems and their solutions.
Problem: The custom context menu doesn’t appear.
Solution: Ensure the id
of the context menu matches the JavaScript selector. Verify CSS settings and make sure display: none;
is removed when intended to be shown.
Problem: Multiple context menus appear on the page.
Solution: Make sure that only one context menu is displayed at a time by hiding any others before showing the new one.
Handling Events Outside the Menu
Another useful feature is to close the context menu when clicking or interacting outside of it.
// Close the context menu on outside click
document.addEventListener('click', function(event) {
if (!menu.contains(event.target)) {
menu.style.display = 'none';
}
});
This snippet ensures that the custom context menu hides when clicking outside it, creating a pleasant user experience.
FAQs Related to Custom Context Menus in JavaScript
How do I make the context menu responsive?
Use CSS media queries to adjust the menu’s style for different screen sizes.
How can I attach events to dynamically created menu items?
Use event delegation by attaching events to a parent element, or reassign event listeners after the menu content updates.
Is it possible to disable specific context menu options?
Yes, you can disable options by adding a class and using CSS or JavaScript to manage their state.
Can I integrate third-party libraries with my custom context menu?
Yes, third-party libraries like jQuery or frameworks like React can work with custom context menus using similar event handling techniques.
How do I ensure my context menu performs well with large datasets?
Implement efficient event handling and consider virtual rendering techniques to handle large data dynamically within the menu.
Shop more on Amazon