Building a Responsive Table with JavaScript
Published June 18, 2024 at 8:25 pm
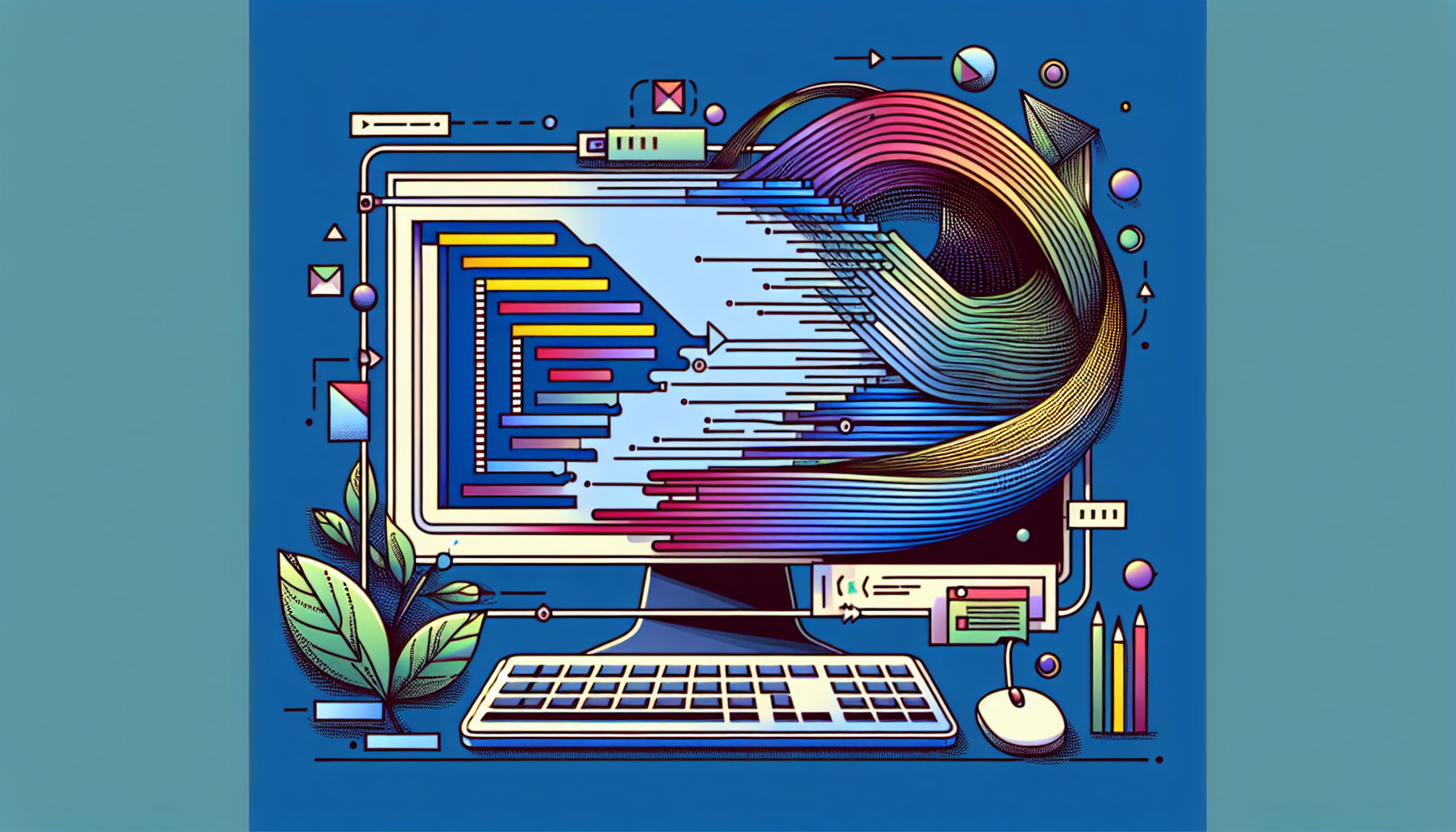
How to Build a Responsive Table with JavaScript
Creating responsive tables in JavaScript can help ensure that your data displays correctly on all devices.
This guide will show you step-by-step how to achieve this using plain JavaScript and CSS.
TL;DR: How to Create a Responsive Table in JavaScript
Here’s a quick solution to build a responsive table using JavaScript and CSS.
// Sample data
const data = [
{ id: 1, name: "John Doe", age: 25 },
{ id: 2, name: "Jane Smith", age: 30 },
{ id: 3, name: "Sam Green", age: 22 },
];
// Function to create the table
function createTable(data) {
const table = document.createElement('table');
const headers = Object.keys(data[0]);
// Create table header
const thead = document.createElement('thead');
const headerRow = document.createElement('tr');
headers.forEach(header => {
const th = document.createElement('th');
th.innerText = header;
headerRow.appendChild(th);
});
thead.appendChild(headerRow);
table.appendChild(thead);
// Create table body
const tbody = document.createElement('tbody');
data.forEach(item => {
const row = document.createElement('tr');
headers.forEach(header => {
const td = document.createElement('td');
td.innerText = item[header];
row.appendChild(td);
});
tbody.appendChild(row);
});
table.appendChild(tbody);
// Append the table to the document
document.body.appendChild(table);
}
// Apply basic CSS for responsiveness
const style = document.createElement('style');
style.innerHTML = `
table {
width: 100%;
border-collapse: collapse;
}
th, td {
padding: 12px;
border: 1px solid #ddd;
text-align: left;
}
@media (max-width: 600px) {
table, thead, tbody, th, td, tr {
display: block;
}
th {
display: none;
}
td {
position: relative;
padding-left: 50%;
}
td::before {
content: attr(data-label);
position: absolute;
left: 0;
width: 50%;
padding: 12px;
white-space: nowrap;
font-weight: bold;
}
}
`;
document.head.appendChild(style);
// Call createTable with sample data
createTable(data);
Introducing Responsive Tables
Responsive tables adapt to different screen sizes seamlessly.
This ensures the data remains readable and accessible on various devices.
Steps to Build a Responsive Table with JavaScript
We will break down each part of the table creation process for better understanding.
Follow these detailed steps to create your own responsive table.
Step 1: Prepare Your Data
Your data should be in an array of objects format.
Each object represents a row in the table.
For example:
const data = [
{ id: 1, name: "John Doe", age: 25 },
{ id: 2, name: "Jane Smith", age: 30 },
{ id: 3, name: "Sam Green", age: 22 },
];
Step 2: Create the Table Header
The table header contains column names.
These are derived from the keys of the first object in the data array.
const headers = Object.keys(data[0]);
const thead = document.createElement('thead');
const headerRow = document.createElement('tr');
headers.forEach(header => {
const th = document.createElement('th');
th.innerText = header;
headerRow.appendChild(th);
});
thead.appendChild(headerRow);
Step 3: Create the Table Body
The table body consists of rows of data.
Each row is an object, and each cell is a value from that object.
const tbody = document.createElement('tbody');
data.forEach(item => {
const row = document.createElement('tr');
headers.forEach(header => {
const td = document.createElement('td');
td.innerText = item[header];
row.appendChild(td);
});
tbody.appendChild(row);
});
Step 4: Assemble the Table
Combine the header and body to form the final table.
You can then append this table to the document.
const table = document.createElement('table');
table.appendChild(thead);
table.appendChild(tbody);
document.body.appendChild(table);
Applying CSS for Responsiveness
Use CSS to make the table responsive.
Here is a basic CSS to achieve this:
const style = document.createElement('style');
style.innerHTML = `
table {
width: 100%;
border-collapse: collapse;
}
th, td {
padding: 12px;
border: 1px solid #ddd;
text-align: left;
}
@media (max-width: 600px) {
table, thead, tbody, th, td, tr {
display: block;
}
th {
display: none;
}
td {
position: relative;
padding-left: 50%;
}
td::before {
content: attr(data-label);
position: absolute;
left: 0;
width: 50%;
padding: 12px;
white-space: nowrap;
font-weight: bold;
}
}
`;
document.head.appendChild(style);
Why Use Responsive Tables?
Responsive tables ensure data is accessible on all devices.
They improve user experience significantly.
Pros and Cons
Responsive tables have their advantages and disadvantages.
Pros:
- Improves accessibility.
- Ensures data is readable on all devices.
- Enhances user experience.
Cons:
- May require extra CSS for complex layouts.
- Can be challenging with very large datasets.
FAQs
How do I make my tables responsive without JavaScript?
Use CSS-only solutions like media queries and flexbox.
Can I use a library to make tables responsive?
Yes, libraries like DataTables can help.
What if my table data is dynamic?
Use JavaScript to update the table when the data changes.
Is it possible to export responsive tables?
Yes, but exporting often requires additional plugins or tools.
How can I handle very large tables?
Consider pagination or lazy loading for performance.
This covers the essentials of creating responsive tables using JavaScript.
Experiment with different approaches to find what works best for your needs.
Your tables will be responsive and user-friendly.
How to Build a Responsive Table with JavaScript
Creating responsive tables in JavaScript can help ensure that your data displays correctly on all devices.
This guide will show you step-by-step how to achieve this using plain JavaScript and CSS.
TL;DR: How to Create a Responsive Table in JavaScript
Here’s a quick solution to build a responsive table using JavaScript and CSS.
// Sample data
const data = [
{ id: 1, name: "John Doe", age: 25 },
{ id: 2, name: "Jane Smith", age: 30 },
{ id: 3, name: "Sam Green", age: 22 },
];
// Function to create the table
function createTable(data) {
const table = document.createElement('table');
const headers = Object.keys(data[0]);
// Create table header
const thead = document.createElement('thead');
const headerRow = document.createElement('tr');
headers.forEach(header => {
const th = document.createElement('th');
th.innerText = header;
headerRow.appendChild(th);
});
thead.appendChild(headerRow);
table.appendChild(thead);
// Create table body
const tbody = document.createElement('tbody');
data.forEach(item => {
const row = document.createElement('tr');
headers.forEach(header => {
const td = document.createElement('td');
td.innerText = item[header];
row.appendChild(td);
});
tbody.appendChild(row);
});
table.appendChild(tbody);
// Append the table to the document
document.body.appendChild(table);
}
// Apply basic CSS for responsiveness
const style = document.createElement('style');
style.innerHTML = `
table {
width: 100%;
border-collapse: collapse;
}
th, td {
padding: 12px;
border: 1px solid #ddd;
text-align: left;
}
@media (max-width: 600px) {
table, thead, tbody, th, td, tr {
display: block;
}
th {
display: none;
}
td {
position: relative;
padding-left: 50%;
}
td::before {
content: attr(data-label);
position: absolute;
left: 0;
width: 50%;
padding: 12px;
white-space: nowrap;
font-weight: bold;
}
}
`;
document.head.appendChild(style);
// Call createTable with sample data
createTable(data);
Introducing Responsive Tables
Responsive tables adapt to different screen sizes seamlessly.
This ensures the data remains readable and accessible on various devices.
Steps to Build a Responsive Table with JavaScript
We will break down each part of the table creation process for better understanding.
Follow these detailed steps to create your own responsive table.
Step 1: Prepare Your Data
Your data should be in an array of objects format.
Each object represents a row in the table.
For example:
const data = [
{ id: 1, name: "John Doe", age: 25 },
{ id: 2, name: "Jane Smith", age: 30 },
{ id: 3, name: "Sam Green", age: 22 },
];
Step 2: Create the Table Header
The table header contains column names.
These are derived from the keys of the first object in the data array.
const headers = Object.keys(data[0]);
const thead = document.createElement('thead');
const headerRow = document.createElement('tr');
headers.forEach(header => {
const th = document.createElement('th');
th.innerText = header;
headerRow.appendChild(th);
});
thead.appendChild(headerRow);
Step 3: Create the Table Body
The table body consists of rows of data.
Each row is an object, and each cell is a value from that object.
const tbody = document.createElement('tbody');
data.forEach(item => {
const row = document.createElement('tr');
headers.forEach(header => {
const td = document.createElement('td');
td.innerText = item[header];
row.appendChild(td);
});
tbody.appendChild(row);
});
Step 4: Assemble the Table
Combine the header and body to form the final table.
You can then append this table to the document.
const table = document.createElement('table');
table.appendChild(thead);
table.appendChild(tbody);
document.body.appendChild(table);
Applying CSS for Responsiveness
Use CSS to make the table responsive.
Here is a basic CSS to achieve this:
const style = document.createElement('style');
style.innerHTML = `
table {
width: 100%;
border-collapse: collapse;
}
th, td {
padding: 12px;
border: 1px solid #ddd;
text-align: left;
}
@media (max-width: 600px) {
table, thead, tbody, th, td, tr {
display: block;
}
th {
display: none;
}
td {
position: relative;
padding-left: 50%;
}
td::before {
content: attr(data-label);
position: absolute;
left: 0;
width: 50%;
padding: 12px;
white-space: nowrap;
font-weight: bold;
}
}
`;
document.head.appendChild(style);
Why Use Responsive Tables?
Responsive tables ensure data is accessible on all devices.
They improve user experience significantly.
Pros and Cons
Responsive tables have their advantages and disadvantages.
Pros:
- Improves accessibility.
- Ensures data is readable on all devices.
- Enhances user experience.
Cons:
- May require extra CSS for complex layouts.
- Can be challenging with very large datasets.
FAQs
How do I make my tables responsive without JavaScript?
Use CSS-only solutions like media queries and flexbox.
Can I use a library to make tables responsive?
Yes, libraries like DataTables can help.
What if my table data is dynamic?
Use JavaScript to update the table when the data changes.
Is it possible to export responsive tables?
Yes, but exporting often requires additional plugins or tools.
How can I handle very large tables?
Consider pagination or lazy loading for performance.
This covers the essentials of creating responsive tables using JavaScript.
Experiment with different approaches to find what works best for your needs.
Your tables will be responsive and user-friendly.
Shop more on Amazon