Creating a Countdown Timer in JavaScript
Published June 25, 2024 at 5:27 pm
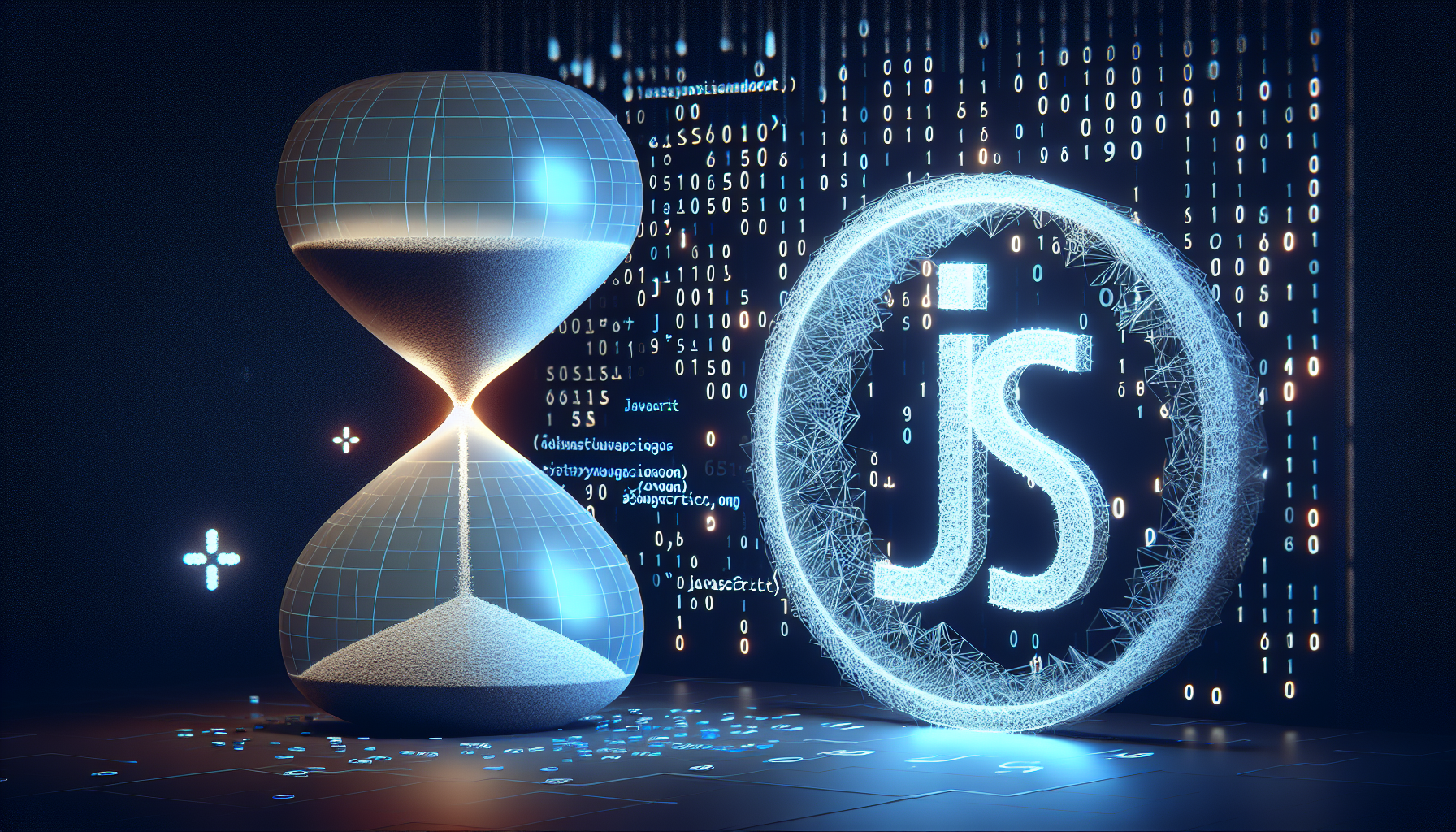
Creating a JavaScript Countdown Timer
A countdown timer is a simple yet useful feature often used in web development..
You might need it for timing events, countdown sales, or any other scenario..
TLDR; How to Create a Countdown Timer in JavaScript
To create a countdown timer, you can use the following JavaScript code snippet.:
//Function to start the countdown timer.
function startCountdown(duration, display) {
var timer = duration;
var minutes, seconds;
setInterval(() => {
minutes = parseInt(timer / 60, 10);
seconds = parseInt(timer % 60, 10);
minutes = minutes < 10 ? "0" + minutes : minutes;
seconds = seconds < 10 ? "0" + seconds : seconds;
display.textContent = minutes + ":" + seconds;
if (--timer < 0) {
timer = duration;
}
}, 1000);
}
window.onload = () => {
var fiveMinutes = 60 * 5;
var display = document.querySelector('#time');
startCountdown(fiveMinutes, display);
};
This JavaScript code creates a countdown timer. It initializes a timer with a duration (in seconds), calculates minutes and seconds, and updates a display every second. Let’s break down the steps.:
Understanding the Code
First, we define the startCountdown
function. This function takes two arguments.:
- duration: The total time of the countdown in seconds.
- display: The HTML element where the countdown will be shown.
Inside the function, we initialize variables.:
var timer = duration;
This stores the countdown duration, which decrements every second.
We also define variables for minutes and seconds. Each second, we recalculate these values and update the display.
The setInterval
method runs the provided function every 1000 milliseconds (1 second).
Next, we use the parseInt
function to convert the duration into minutes and seconds. If the values are less than ten, we prepend a zero to maintain a two-digit format.
Finally, we update the text content of the display element each second, showing the remaining time. When the timer reaches zero, it resets to the original duration. This loop will continue indefinitely. For a one-time countdown, you can modify the code to stop the interval when the timer hits zero.
Adding Countdown Timer to Your Web Page
To integrate the countdown timer into your web page, you need an HTML element to display the timer. Here’s an example.:
In this example, we have a div
element with a span
inside it. The span
element has an id of “time”, which is used by our JavaScript code to update the remaining time.
Customizing the Countdown Timer
Depending on your needs, you may want to customize the countdown timer further. Here are some ideas.:
- Changing the initial duration.
- Adding more styling to the timer display.
- Triggering an event when the countdown reaches zero.
Example: Changing the Initial Duration
If you want to change the starting time of your countdown timer, simply modify the fiveMinutes
variable. For instance, to set a 10-minute timer.:
var tenMinutes = 60 * 10;
var display = document.querySelector('#time');
startCountdown(tenMinutes, display);
Example: Adding More Styling
You can add CSS to style your countdown timer. Below is an example.:
This CSS makes the countdown timer text larger and changes its color to red. You can customize it further to match your website’s design.
Handling Countdown Completion
Once the countdown reaches zero, you might want to trigger an event, such as displaying a message or redirecting the user. To do this, modify the startCountdown
function to include your desired action.:
function startCountdown(duration, display) {
var timer = duration, minutes, seconds;
var interval = setInterval(() => {
minutes = parseInt(timer / 60, 10);
seconds = parseInt(timer % 60, 10);
minutes = minutes < 10 ? "0" + minutes : minutes; seconds = seconds < 10 ? "0" + seconds : seconds; display.textContent = minutes + ":" + seconds; if (--timer < 0) { clearInterval(interval); display.textContent = "Time's up!"; } }, 1000); }
In this modified version, we use the clearInterval
function to stop the interval when the timer reaches zero. We then change the display text to "Time's up!".
Common Issues and Troubleshooting
Here are some common issues you might face while creating a countdown timer and how to fix them.:
Countdown Timer Not Updating
If your countdown timer isn't updating, ensure the following.:
- The
setInterval
method is correctly called. - The HTML element with the specified id exists.
- There are no JavaScript errors in the console.
Countdown Timer Resets Immediately
If the timer resets immediately, check the condition inside the setInterval
function..
Ensure you decrement the timer correctly and reset it only when it reaches zero.
Styling Not Applied
If your styles aren't applied, ensure the CSS is correctly linked. Check for any typos or syntax errors in your CSS code.
FAQs
How do I pause and resume the countdown timer?
To pause and resume the countdown timer, you'll need to keep track of the timer state. You can modify the startCountdown
function to allow pausing and resuming.:
var timer;
var isPaused = false;
var interval;
function startCountdown(duration, display) {
timer = duration;
interval = setInterval(() => {
if (!isPaused) {
var minutes = parseInt(timer / 60, 10);
var seconds = parseInt(timer % 60, 10);
minutes = minutes < 10 ? "0" + minutes : minutes; seconds = seconds < 10 ? "0" + seconds : seconds; display.textContent = minutes + ":" + seconds; if (--timer < 0) { clearInterval(interval); display.textContent = "Time's up!"; } } }, 1000); } function pauseTimer() { isPaused = true; } function resumeTimer() { isPaused = false; }
Can I format the timer to show hours and minutes?
Yes, you can. Modify the startCountdown
function to include hours.:
function startCountdown(duration, display) {
var timer = duration;
var hours, minutes, seconds;
setInterval(() => {
hours = parseInt(timer / 3600, 10);
minutes = parseInt((timer % 3600) / 60, 10);
seconds = parseInt(timer % 60, 10);
hours = hours < 10 ? "0" + hours : hours; minutes = minutes < 10 ? "0" + minutes : minutes; seconds = seconds < 10 ? "0" + seconds : seconds; display.textContent = hours + ":" + minutes + ":" + seconds; if (--timer < 0) { timer = duration; } }, 1000); }
How can I add sound notifications to the timer?
You can add sound notifications by playing an audio file when the timer reaches zero. Here's an example.:
function startCountdown(duration, display) {
var timer = duration;
var minutes, seconds;
var audio = new Audio('path_to_audio_file.mp3');
setInterval(() => {
minutes = parseInt(timer / 60, 10);
seconds = parseInt(timer % 60, 10);
minutes = minutes < 10 ? "0" + minutes : minutes; seconds = seconds < 10 ? "0" + seconds : seconds; display.textContent = minutes + ":" + seconds; if (--timer < 0) { audio.play(); timer = duration; } }, 1000); }
This guide has walked through creating a countdown timer in JavaScript with practical examples.
Enhancing Your Countdown Timer with Additional Features
Now that you have a basic countdown timer, let's explore some additional features to make it even more useful and engaging.
Adding a Start and Reset Button
To provide more control over the countdown timer, you can add start and reset buttons. Here's how you can do it.:
This example adds two buttons for starting and resetting the timer. The startTimer
function starts the countdown, and the resetTimer
function resets it to the original duration.
Adding a Countdown Pause and Resume Feature
You might want to give users the ability to pause and resume the countdown. Here's how you can implement that.:
In this code, we track the pause state using the isPaused
variable. The pauseTimer
function sets this variable to true, while the resumeTimer
function sets it to false. The setInterval
callback checks the isPaused
state before updating the timer.
Using the Countdown Timer with Custom Events
You might want to add custom events when the countdown reaches a certain time. You can do this by modifying the countdown logic.:
function startCountdown(duration, display) {
var timer = duration;
var minutes, seconds;
var halfway = duration / 2;
var almostEnd = duration / 10;
var interval = setInterval(() => {
minutes = parseInt(timer / 60, 10);
seconds = parseInt(timer % 60, 10);
minutes = minutes < 10 ? "0" + minutes : minutes; seconds = seconds < 10 ? "0" + seconds : seconds; display.textContent = minutes + ":" + seconds; if (timer == halfway) { alert("Halfway there!"); } else if (timer == almostEnd) { alert("Almost done!"); } if (--timer < 0) { clearInterval(interval); display.textContent = "Time's up!"; } }, 1000); }
In this example, we define custom alerts when the timer reaches halfway and almost ending points. You can replace alert
with any desired custom action.
Setting Up Multiple Countdown Timers on One Page
You might need to run multiple countdown timers on the same web page. Here is an example of setting up more than one timer.:
In this example, we set up two countdown timers on a single web page. Each timer runs independently of the other.
Advanced Customizations for Your Countdown Timer
If you need more advanced customizations, like animations or progress bars, consider integrating with JavaScript libraries. Libraries like jQuery and frameworks like React or Vue can make complex features easier to implement.
This guide explained how to create a countdown timer in JavaScript and enhance it with various features.
FAQs
How do I change the countdown format to include days?
To include days in your countdown timer, modify the startCountdown
function to calculate and display days.:
function startCountdown(duration, display) {
var timer = duration;
var days, hours, minutes, seconds;
setInterval(() => {
days = parseInt(timer / 86400, 10);
hours = parseInt((timer % 86400) / 3600, 10);
minutes = parseInt((timer % 3600) / 60, 10);
seconds = parseInt(timer % 60, 10);
days = days < 10 ? "0" + days : days; hours = hours < 10 ? "0" + hours : hours; minutes = minutes < 10 ? "0" + minutes : minutes; seconds = seconds < 10 ? "0" + seconds : seconds; display.textContent = days + ":" + hours + ":" + minutes + ":" + seconds; if (--timer < 0) { clearInterval(interval); display.textContent = "Time's up!"; } }, 1000); }
How can I save the countdown state between page reloads?
You can save the state using localStorage.:
function startCountdown(duration, display) {
var timer = duration;
if (localStorage.getItem('countdown')) {
timer = localStorage.getItem('countdown');
}
setInterval(() => {
var minutes = parseInt(timer / 60, 10);
var seconds = parseInt(timer % 60, 10);
minutes = minutes < 10 ? "0" + minutes : minutes; seconds = seconds < 10 ? "0" + seconds : seconds; display.textContent = minutes + ":" + seconds; localStorage.setItem('countdown', timer); if (--timer < 0) { clearInterval(interval); display.textContent = "Time's up!"; localStorage.removeItem('countdown'); } }, 1000); }
Can I create multiple timers on different pages with sync?
To sync multiple timers across different pages, use a backend server or a cloud-based database like Firebase.
Shop more on Amazon