How to Implement Debounce and Throttle in JavaScript
Published June 29, 2024 at 3:34 pm
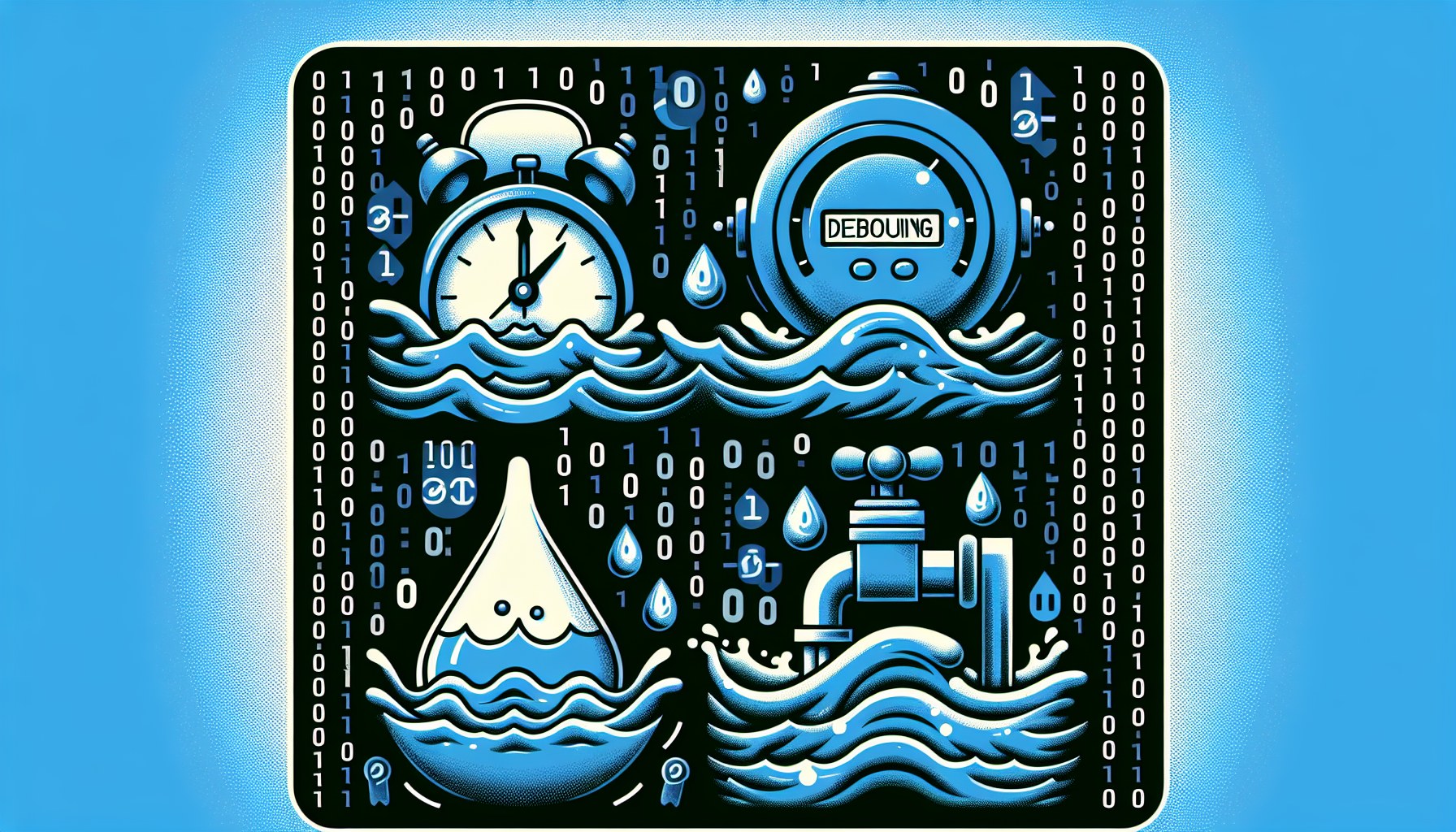
What is Debounce and Throttle in JavaScript?
In JavaScript, debounce and throttle are techniques to control how often a function is executed.
They are particularly useful for improving the performance of web applications by limiting the rate at which functions are called.
**Debounce:** Only allow a function to be executed after a specified delay from the last call.
**Throttle:** Ensure a function is called at most once within a specified time period.
TLDR: How to Implement Debounce and Throttle in JavaScript
**Debounce:**
function debounce(func, wait) {
let timeout;
return function(...args) {
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(this, args), wait);
};
}
**Throttle:**
function throttle(func, limit) {
let inThrottle;
return function(...args) {
if (!inThrottle) {
func.apply(this, args);
inThrottle = true;
setTimeout(() => (inThrottle = false), limit);
}
};
}
Why and When to Use Debounce and Throttle
Debounce and throttle are essential to enhance user experience and performance.
They are crucial when dealing with events that fire frequently, such as scrolling or resizing.
Scenarios for Debounce Beneficial:
- Form validation as you type
- Search input with real-time suggestions
Scenarios for Throttle Beneficial:
- Scroll event handling
- Resizing a window
- Preventing button click spamming
Implementing Debounce in JavaScript
Let’s start with debounce. It ensures a function is delayed until after a specified wait time has passed since the last time it was invoked.
This is particularly useful in scenarios where you only want the function to execute after the user has stopped performing an action.
**Example:** Using debounce to handle a search input:
const searchInput = document.getElementById('searchInput');
const handleSearch = debounce(function(event) {
console.log('Searching: ', event.target.value);
}, 500);
searchInput.addEventListener('input', handleSearch);
// The search function will be called 500ms after the user stops typing.
In the code above, the handleSearch function is executed only after the user has stopped typing for 500 milliseconds.
This reduces the number of times the search function is called, improving performance.
Implementing Throttle in JavaScript
Throttle, on the other hand, ensures that a function is called at most once in a specified time interval.
This is useful in scenarios where you want a continuous action but at a controlled rate.
**Example:** Using throttle to handle window resizing:
window.addEventListener('resize', throttle(() => {
console.log('Resizing...');
}, 1000));
// The resize event handler is called at most once every second.
In this example, the resizing function is called at most once every second, preventing performance issues caused by continuously firing resize events.
Combining Debounce and Throttle
There could be scenarios where you might need to combine debounce and throttle.
This can provide you with more control over how frequently your functions execute.
**Example:** Advanced search input field:
const searchInput = document.getElementById('searchInput');
const handleInputChange = debounce(throttle(function(event) {
console.log('Advanced Search: ', event.target.value);
}, 1000), 300);
searchInput.addEventListener('input', handleInputChange);
// This approach will delay input handling by 300ms and will handle it at most every second.
In this example, the search function is debounced by 300 milliseconds and throttled to execute at most once every second.
This combination can help optimize performance while still providing a responsive user experience.
Pros and Cons of Debounce and Throttle
Pros of Debounce:
- Improves performance by limiting function calls.
- Useful for input validation and search functionalities.
Cons of Debounce:
- May cause delays in user feedback.
- Not suitable for continuous events like scrolling.
Pros of Throttle:
- Ensures functions are called at controlled intervals.
- Ideal for continuous events like scrolling and resizing.
Cons of Throttle:
- May miss some function calls if the interval is too long.
- Can make real-time applications feel less responsive.
Frequently Asked Questions
What is the difference between debounce and throttle?
Debounce delays function execution until after a specified time since the last call.
Throttle ensures a function is called at most once within a specified time period.
When should I use debounce?
Use debounce for actions that do not need to happen every time an event fires, like a search input.
When should I use throttle?
Use throttle for continuous events that need to be controlled, like resizing or scrolling.
Can debounce and throttle be combined?
Yes, they can be combined to provide more control over function execution.
What are common use cases for debounce?
Form validation, search inputs, and button click handling.
What are common use cases for throttle?
Scroll events, resize events, and API requests.
Understanding Real-World Examples of Debounce and Throttle
In real-world applications, debounce and throttle can significantly improve performance and user experience.
Let’s look at more examples to better understand their application.
**Example:** Image gallery with infinite scroll using throttle:
window.addEventListener('scroll', throttle(() => {
if (window.innerHeight + window.scrollY >= document.body.offsetHeight) {
loadMoreImages();
}
}, 2000));
// Load more images at most once every 2 seconds when the user scrolls to the bottom.
In this example, the loadMoreImages function is throttled to execute at most once every 2 seconds while the user scrolls.
This prevents performance issues due to frequent scroll events.
Common Mistakes and How to Avoid Them
When implementing debounce and throttle, certain mistakes are common.
**Mistake:** Using debounced inputs in scenarios requiring immediate feedback.
**Solution:** Use throttle instead to provide immediate feedback at controlled intervals.
**Mistake:** Setting the throttle or debounce interval too high.
**Solution:** Test different intervals to find a balance between performance and responsiveness.
Exploring JavaScript Libraries for Debounce and Throttle
Several JavaScript libraries offer built-in debounce and throttle functions.
Lodash is a popular utility library that includes these functionalities.
**Using Lodash:**
const _ = require('lodash');
// Lodash debounce example
const debouncedFunction = _.debounce(() => {
console.log('Debounced function called');
}, 300);
debouncedFunction();
// Lodash throttle example
const throttledFunction = _.throttle(() => {
console.log('Throttled function called');
}, 1000);
throttledFunction();
Lodash provides a reliable and easy way to implement debounce and throttle in your projects.
Wrapping Up
Understanding and implementing debounce and throttle in JavaScript helps improve web application performance.
By controlling how often functions are executed, you can enhance the user experience without compromising on responsiveness.
Implementing Debounce in Real-World Scenarios
It’s time to dive deeper into how to use debounce in actual projects.
This section will show how to apply debounce to different functions to improve your web application’s performance.
**Example:** Debounce for form validation:
const validateForm = debounce(function() {
// Form validation logic goes here
console.log('Form validated');
}, 1000);
document.getElementById('myForm').addEventListener('input', validateForm);
// Validate form 1 second after user stops typing.
Applying debounce to form validation can significantly improve performance.
It ensures validation runs only once after the user finishes typing.
Implementing Throttle in Real-World Scenarios
Throttle can be a lifesaver in various scenarios where continuous execution can cause performance issues.
Below, we explore more ways to use throttle.
**Example:** Throttle for a scroll event that updates an element’s visibility:
const checkVisibility = throttle(function() {
const element = document.getElementById('myElement');
if (window.scrollY >= 200) {
element.style.visibility = 'visible';
} else {
element.style.visibility = 'hidden';
}
}, 300);
document.addEventListener('scroll', checkVisibility);
// Check visibility state every 300ms while scrolling.
This approach ensures the visibility check happens at controlled intervals.
Combining Advanced Debounce and Throttle Techniques
In certain advanced scenarios, combining debounce and throttle might yield optimal results.
This combination is rarely needed but can be very effective.
**Example:** Real-time chat application with debounce and throttle:
const messageInput = document.getElementById('messageInput');
const sendMessage = debounce(throttle(function(event) {
console.log('Sending message:', event.target.value);
}, 200), 300);
messageInput.addEventListener('input', sendMessage);
// Send message at most every 200ms, but with a delay of 300ms after user stops typing.
This scenario helps manage message sending functionality in a chat application.
Exploring JavaScript Libraries for Debounce and Throttle Range
Many JavaScript libraries can help simplify the implementation of debounce and throttle.
Lodash is one of the most popular libraries that offer these functionalities.
We’ve already provided a basic overview of how to use Lodash earlier.
Here, we can delve a bit deeper into more advanced use cases.
**Example:** Using Lodash for a dynamic resize and scroll event:
const _ = require('lodash');
// Lodash debounce for resize event
const handleResize = _.debounce(() => {
console.log('Window resized');
}, 500);
window.addEventListener('resize', handleResize);
// Lodash throttle for scroll event
const handleScroll = _.throttle(() => {
console.log('Window scrolled');
}, 200);
window.addEventListener('scroll', handleScroll);
These examples demonstrate advanced use cases using Lodash.
Key Differences Between Debounce and Throttle
It’s essential to recap the main differences between debounce and throttle.
Understanding these differences helps you make informed decisions about which to use in various situations.
**Debounce:** Delays execution until a certain amount of time has passed since the last call.
**Throttle:** Ensures the function is called at most once within a specified period.
Frequently Asked Questions
Can I use debounce and throttle together?
Yes, combining them can help control function execution more effectively.
Is Lodash the best library for debounce and throttle?
Lodash is widely used but other libraries like underscore.js also offer similar functions.
Do debounce and throttle affect performance?
Yes, they can significantly enhance performance by reducing the number of function executions.
What is a common mistake when implementing debounce?
Setting the delay too long can result in poor user experience.
How does throttle improve user experience?
Throttle ensures functions are executed at balanced intervals, providing smoother experiences.
Advanced Techniques for Debugging Debounce and Throttle
Sometimes, implementing debounce and throttle might not work as expected.
Here are some advanced debugging techniques for these scenarios.
**Console Logging:** Add console logs to understand the execution flow.
**Example:**
function throttle(func, limit) {
let inThrottle;
return function(...args) {
console.log('Throttle called');
if (!inThrottle) {
func.apply(this, args);
inThrottle = true;
setTimeout(() => {
inThrottle = false;
console.log('Throttle reset');
}, limit);
}
};
}
This example shows how to add logging to understand the throttle flow.
**Network Tab:** Use the browser’s network tab to analyze API requests.
Ensure that the requests reflect the debounce or throttle intervals.
Best Practices for Using Debounce and Throttle
Adhering to best practices can elevate your use of debounce and throttle.
Here are some tips to get the most out of these techniques.
**Determine Necessity:** Only use debounce or throttle when needed.
Adding them to every function can lead to unnecessary complexity.
**Appropriate Delays:** Test various intervals to find the balance.
Avoid setting extremely long or short delays.
**Use Libraries:** Leveraging libraries like Lodash can save time.
They provide well-tested implementations of debounce and throttle.
Ensuring Compatibility Across Browsers
Compatibility is crucial for web applications targeting multiple browsers.
Debounce and throttle functions should be thoroughly tested for cross-browser compatibility.
One approach is to use polyfills or libraries that ensure cross-browser support.
Most modern libraries take these factors into account and offer reliable solutions.
Custom Implementations and Extensions
Sometimes, you might need custom implementations of debounce and throttle.
Creating these tailored solutions allows for unique handling scenarios specific to your needs.
**Example:** Creating a throttled debounce function:
function throttledDebounce(func, wait, throttleLimit) {
let timeout;
let lastCall = 0;
return function(...args) {
const now = new Date().getTime();
if (now - lastCall > throttleLimit) {
func.apply(this, args);
lastCall = now;
} else {
clearTimeout(timeout);
timeout = setTimeout(() => {
func.apply(this, args);
lastCall = now;
}, wait);
}
};
}
const customFunction = throttledDebounce(() => {
console.log('Custom function called');
}, 300, 1000);
customFunction();
This custom implementation combines the benefits of both debounce and throttle.
The code ensures that the function is called not too frequently.
Conclusion and What We Learned
Understanding debounce and throttle is essential for optimizing web applications.
Implementing these techniques ensures better performance and improved user experiences.
We explored various ways to use debounce and throttle in JavaScript.
Applying these methods equips you with tools to handle event-driven scenarios effectively.
Shop more on Amazon