Implementing Dark Mode with JavaScript
Published June 28, 2024 at 6:11 pm
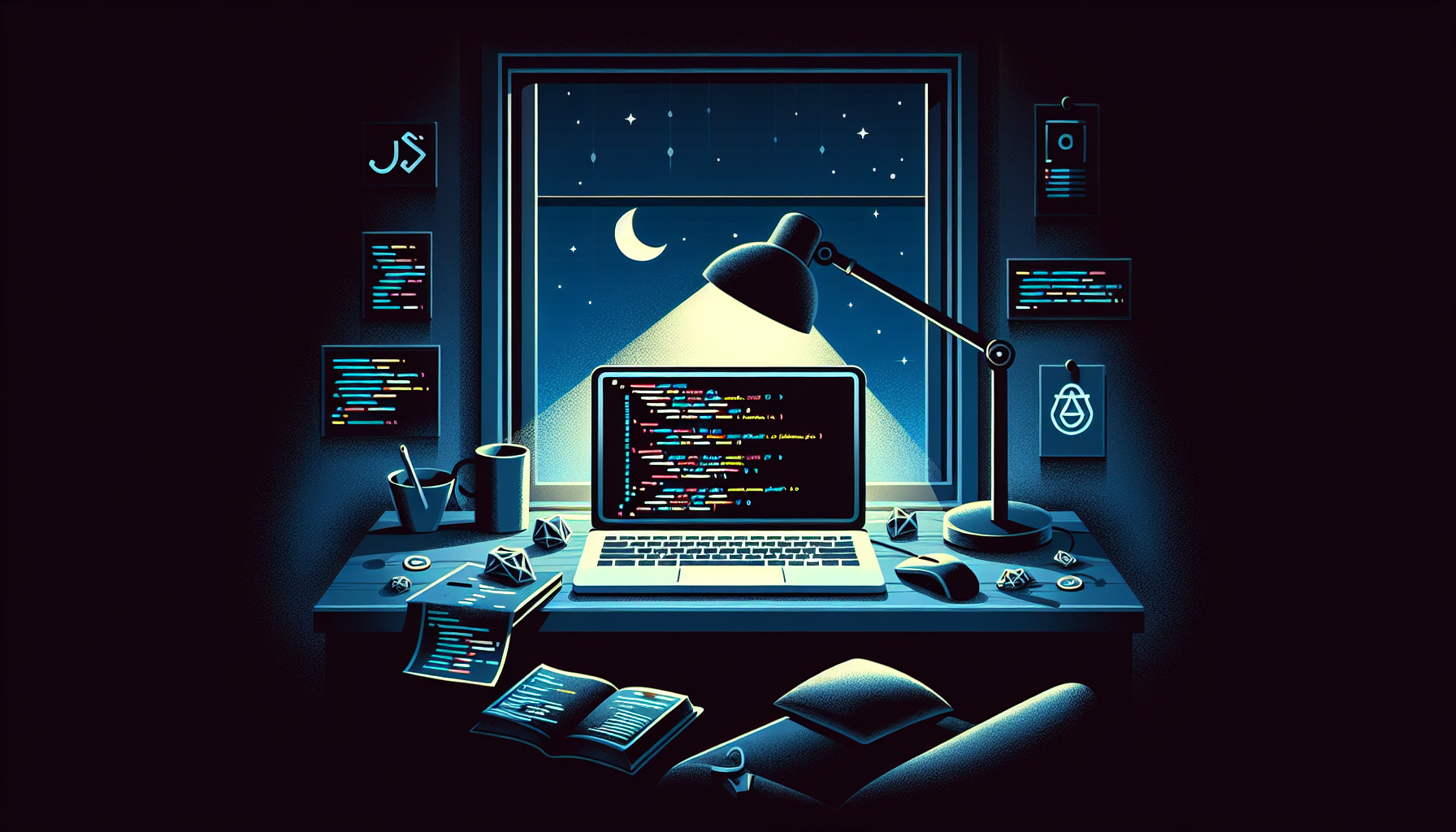
Why Implement Dark Mode in Your Website?
Dark mode is not just a trendy feature; it provides real benefits for users.
It can reduce eye strain, save battery life on devices with OLED screens, and improve accessibility for users with visual impairments.
Incorporating dark mode into your website can enhance the user experience significantly.
By using JavaScript, you can implement a dynamic dark mode that allows users to switch between light and dark themes easily.
TL;DR: How to Implement Dark Mode with JavaScript
Here is a quick solution using JavaScript to implement dark mode in your website:
// Detect user's system preference for dark mode
const userPrefersDark = window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches;
// Initial theme setup based on user preference
document.addEventListener('DOMContentLoaded', (event) => {
const storedTheme = localStorage.getItem('theme');
if (storedTheme) {
document.documentElement.setAttribute('data-theme', storedTheme);
} else if (userPrefersDark) {
document.documentElement.setAttribute('data-theme', 'dark');
}
});
// Function to toggle between dark and light themes
function toggleTheme() {
const currentTheme = document.documentElement.getAttribute('data-theme');
if (currentTheme === 'dark') {
document.documentElement.setAttribute('data-theme', 'light');
localStorage.setItem('theme', 'light');
} else {
document.documentElement.setAttribute('data-theme', 'dark');
localStorage.setItem('theme', 'dark');
}
}
// Event listener to switch themes on button click
document.getElementById('theme-toggle-btn').addEventListener('click', toggleTheme);
Step-by-Step Guide to Implementing Dark Mode
1. Setting Up Your HTML and CSS
The first step in implementing dark mode is to add the necessary HTML and CSS.
Here’s how you can set it up:
2. Adding JavaScript for Theme Toggling
Next, add JavaScript to handle the theme toggling functionality.
Below is a complete JavaScript code example explaining each part:
Dealing with Browser Compatibility
Browser compatibility can be a concern when implementing dark mode.
Most modern browsers support the prefers-color-scheme
media query.
Always test your dark mode implementation on multiple browsers to ensure consistent behavior.
Dark Mode Activation Based on System Preferences
To make the dark mode experience more seamless, you can automatically activate dark mode based on the user’s system preferences.
Here’s how to do it:
const userPrefersDark = window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches;
This code checks if the user’s system is set to dark mode.
If it is, you can set your website to dark mode by default.
Storing User Preferences
Using local storage, you can remember the user’s theme preference.
This ensures that when they return to your site, it displays their preferred theme.
const storedTheme = localStorage.getItem('theme');
if (storedTheme) {
document.documentElement.setAttribute('data-theme', storedTheme);
}
Advanced Customization
You can add more customization options for dark mode.
For example, you can change the color of specific elements or add animations when switching themes.
Here’s an example:
Common Issues and Troubleshooting
While implementing dark mode, you might encounter some common issues.
Below are a few problems and how to fix them:
Issue: Theme not saving after page reload
Ensure you are using local storage correctly to save the user’s preference.
Issue: Colors not changing for all elements
Make sure all elements use CSS variables for their colors.
This ensures that the theme toggle affects all elements.
Issue: Browser compatibility issues
Test your implementation on various browsers to ensure compatibility.
Also, consider providing a fallback in case the prefers-color-scheme
media query is not supported.
Frequently Asked Questions
How can I automatically detect the user’s system theme?
You can use the prefers-color-scheme
media query to detect the user’s system theme.
Can I use dark mode with CSS only?
Yes, but using JavaScript provides a more dynamic experience for users.
Is it necessary to save the user’s theme preference?
Saving the user’s preference ensures a consistent experience every time they visit your site.
What are the advantages of using CSS variables?
CSS variables make it easier to manage and change theme-related styles dynamically.
This simplifies the process of switching between light and dark themes.
Why Implement Dark Mode in Your Website?
Dark mode is not just a trendy feature; it provides real benefits for users.
It can reduce eye strain, save battery life on devices with OLED screens, and improve accessibility for users with visual impairments.
Incorporating dark mode into your website can enhance the user experience significantly.
By using JavaScript, you can implement a dynamic dark mode that allows users to switch between light and dark themes easily.
TL;DR: How to Implement Dark Mode with JavaScript
Here is a quick solution using JavaScript to implement dark mode in your website:
// Detect user's system preference for dark mode
const userPrefersDark = window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches;
// Initial theme setup based on user preference
document.addEventListener('DOMContentLoaded', (event) => {
const storedTheme = localStorage.getItem('theme');
if (storedTheme) {
document.documentElement.setAttribute('data-theme', storedTheme);
} else if (userPrefersDark) {
document.documentElement.setAttribute('data-theme', 'dark');
}
});
// Function to toggle between dark and light themes
function toggleTheme() {
const currentTheme = document.documentElement.getAttribute('data-theme');
if (currentTheme === 'dark') {
document.documentElement.setAttribute('data-theme', 'light');
localStorage.setItem('theme', 'light');
} else {
document.documentElement.setAttribute('data-theme', 'dark');
localStorage.setItem('theme', 'dark');
}
}
// Event listener to switch themes on button click
document.getElementById('theme-toggle-btn').addEventListener('click', toggleTheme);
Step-by-Step Guide to Implementing Dark Mode
Setting Up Your HTML and CSS
The first step in implementing dark mode is to add the necessary HTML and CSS.
Here’s how you can set it up:
Adding JavaScript for Theme Toggling
Next, add JavaScript to handle the theme toggling functionality.
Below is a complete JavaScript code example explaining each part:
Dealing with Browser Compatibility
Browser compatibility can be a concern when implementing dark mode.
Most modern browsers support the prefers-color-scheme
media query.
Always test your dark mode implementation on multiple browsers to ensure consistent behavior.
Dark Mode Activation Based on System Preferences
To make the dark mode experience more seamless, you can automatically activate dark mode based on the user’s system preferences.
Here’s how to do it:
const userPrefersDark = window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches;
This code checks if the user’s system is set to dark mode.
If it is, you can set your website to dark mode by default.
Storing User Preferences
Using local storage, you can remember the user’s theme preference.
This ensures that when they return to your site, it displays their preferred theme.
const storedTheme = localStorage.getItem('theme');
if (storedTheme) {
document.documentElement.setAttribute('data-theme', storedTheme);
}
Advanced Customization
You can add more customization options for dark mode.
For example, you can change the color of specific elements or add animations when switching themes.
Here’s an example:
Common Issues and Troubleshooting
While implementing dark mode, you might encounter some common issues.
Below are a few problems and how to fix them:
Issue: Theme not saving after page reload
Ensure you are using local storage correctly to save the user’s preference.
Issue: Colors not changing for all elements
Make sure all elements use CSS variables for their colors.
This ensures that the theme toggle affects all elements.
Issue: Browser compatibility issues
Test your implementation on various browsers to ensure compatibility.
Also, consider providing a fallback in case the prefers-color-scheme
media query is not supported.
Frequently Asked Questions
How can I automatically detect the user’s system theme?
You can use the prefers-color-scheme
media query to detect the user’s system theme.
Can I use dark mode with CSS only?
Yes, but using JavaScript provides a more dynamic experience for users.
Is it necessary to save the user’s theme preference?
Saving the user’s preference ensures a consistent experience every time they visit your site.
What are the advantages of using CSS variables?
CSS variables make it easier to manage and change theme-related styles dynamically.
This simplifies the process of switching between light and dark themes.
Shop more on Amazon