JavaScript and CSS Transitions: Adding Animations
Published June 24, 2024 at 5:34 pm
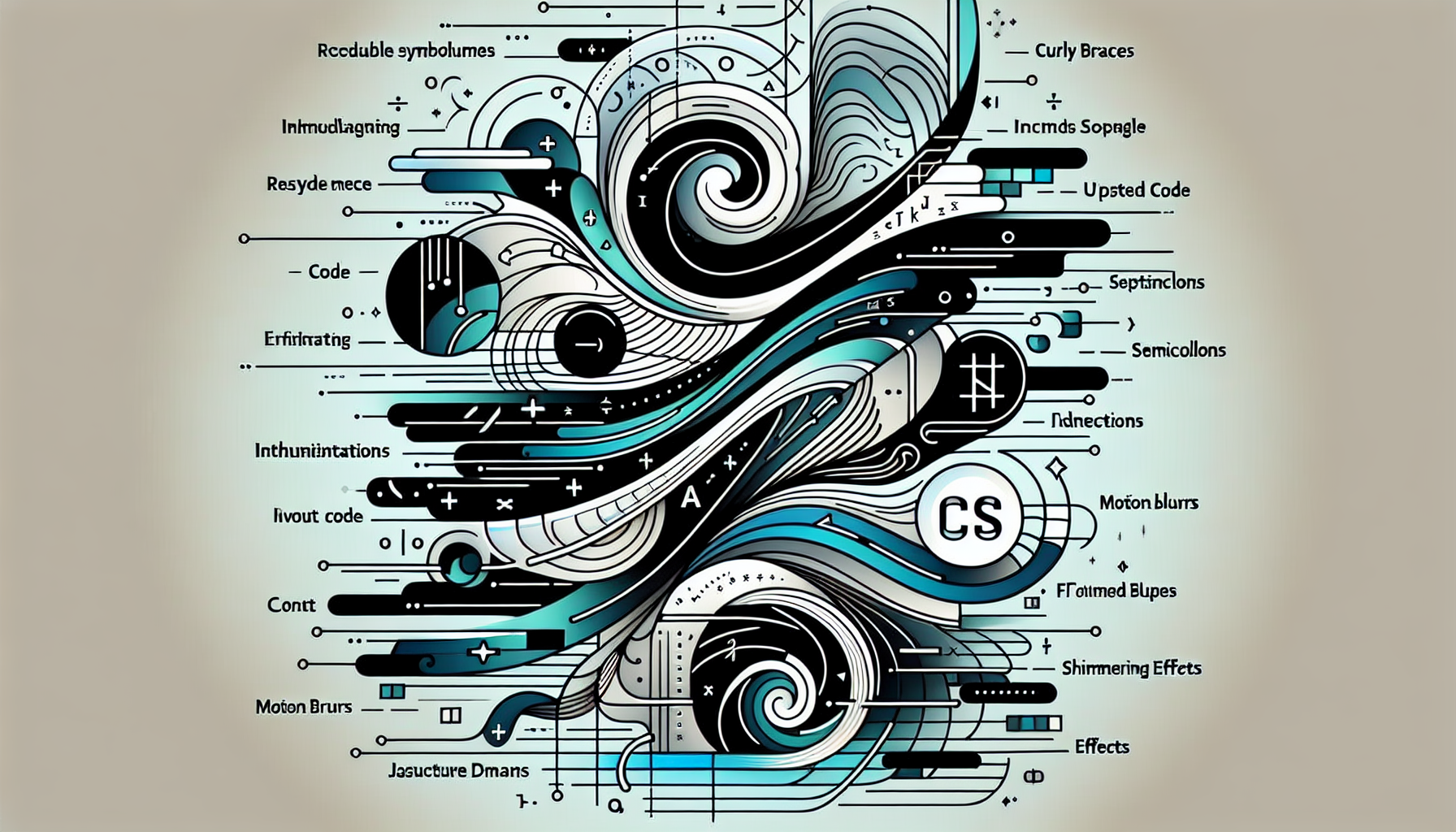
JavaScript and CSS Transitions: Adding Animations
Building interactive and visually appealing web applications might be on your priority list as a developer. Adding animations can significantly uplift the user experience of your website. JavaScript and CSS transitions are two powerful tools that can help you achieve this.
The easiest way to add animations to your web application is to use CSS transitions. You can add a transition effect to any CSS property, such as color, width, height, and more.
For a quick example, you can use:
.element {
width: 100px;
height: 100px;
background-color: red;
transition: width 2s, height 2s, background-color 2s;
}
.element:hover {
width: 200px;
height: 200px;
background-color: blue;
}
What Are CSS Transitions?
CSS transitions allow you to change property values smoothly over a specified duration. With CSS, you can easily create effects when CSS properties change state without having to use JavaScript.
CSS transitions are defined by specifying the duration and the property to animate. They are typically used for hover effects, focus effects, and more.
How to Implement CSS Transitions?
To implement CSS transitions, you can define it in your CSS file. Here’s a basic example:
.button {
background-color: lightblue;
transition: background-color 0.5s ease;
}
.button:hover {
background-color: lightgreen;
}
Understanding Key Properties of CSS Transitions
There are several key properties associated with CSS transitions. These properties allow you to define how, when, and what to animate. These are:
- transition-property: The CSS property you want to animate.
- transition-duration: The time it should take for the transition to complete.
- transition-timing-function: The speed curve of the transition effect.
- transition-delay: Time to wait before starting the transition effect.
Using CSS Keyframes for More Complex Animations
For more complex animations, you might want to use CSS keyframes. Keyframes provide more control over intermediate steps in an animation sequence.
Here’s an example of a simple CSS keyframe animation:
@keyframes example {
from {background-color: red;}
to {background-color: yellow;}
}
.element {
animation-name: example;
animation-duration: 4s;
}
JavaScript Transitions for Enhanced Control
At times, CSS might not be enough to achieve the desired animation effects. This is where JavaScript comes into play. JavaScript provides greater control and can trigger animations in response to more complex events.
Here’s an example of a JavaScript transition on a button click:
document.getElementById("animateBtn").onclick = function() {
let element = document.getElementById("box");
element.style.transition = "width 2s, height 2s, background-color 2s";
element.style.width = "200px";
element.style.height = "200px";
element.style.backgroundColor = "blue";
}
Combining CSS and JavaScript for Robust Animations
Combining CSS transitions and JavaScript can help create more dynamic and robust animations. Use JavaScript to add, remove or toggle classes that control CSS transitions.
For example:
Troubleshooting Common CSS Transition Issues
Sometimes, CSS transitions might not work as expected. Here are some common issues and how to fix them.
Incorrect Property Name:
Ensure the property name you’re trying to animate is correct. Double-check for typos.
Duration Not Set:
Transitions won’t work if you haven’t set a duration. Ensure you specify `transition-duration`.
Transition Not Applied:
The transition property might not apply if there is no initial state defined to transition from.
FAQs
How do you create a basic CSS transition?
Use the `transition` property along with the desired CSS property, duration, timing function, and delay.
Can I control animations using JavaScript?
Yes, JavaScript can dynamically change CSS properties to create animations.
What is the purpose of the `transition-timing-function`?
This property defines the speed curve of the transition effect.
Can CSS transitions and keyframes be combined?
Yes, combining both can create more complex and smooth animations.
What are keyframes in CSS?
Keyframes define the intermediate steps in a CSS animation sequence.
Practical Examples of CSS Transitions
To better understand CSS transitions, let’s dive into some practical examples. This will give you a clearer idea of how to implement them in real-world scenarios.
Basic Fade-In Effect:
You might want to create a smooth fade-in effect for a div element. Here’s how you can do it:
.fade-in {
opacity: 0;
transition: opacity 1.5s ease-in-out;
}
.fade-in:hover {
opacity: 1;
}
In the example above, the `opacity` of the element transitions from 0 to 1 over a duration of 1.5 seconds whenever the element is hovered over.
Sliding Menu Example:
Let’s consider a scenario where you want a menu to slide in from the left when a button is clicked. Here’s a basic implementation:
In this example, clicking the button toggles the `open` class on the menu, causing it to smoothly transition between a width of 0 and 200px.
JavaScript Libraries for Animations
While CSS transitions and JavaScript can achieve a lot on their own, sometimes you might need more advanced animations. This is where JavaScript libraries come in handy.
Using Anime.js:
Anime.js is a powerful animation library for JavaScript. It offers a wide variety of methods to create complex animations. Here’s an example of how you can animate an element using Anime.js:
In this example, the `.box` element translates 250 pixels to the right over a duration of 800 milliseconds with an ‘easeInOutQuad’ easing function.
Using GreenSock (GSAP):
GreenSock Animation Platform (GSAP) is another popular library for animating elements. Here’s a basic example:
Here, the `.circle` element moves 100 pixels to the right, changes its background color to blue, and becomes a circle over a duration of 2 seconds using the ‘power1.inOut’ easing function.
Enhancing User Experience with Animations
Animations are not just for aesthetics; they can significantly enhance the user experience by providing visual feedback, guiding users, and adding a feel of responsiveness to your web application.
Visual Feedback:
Animations can be used to provide visual feedback to users, such as highlighting form fields in error, or drawing attention to important elements.
Smoother Navigation:
Adding animations can make page transitions smoother, helping users feel more comfortable as they navigate through your content.
Engaging Interactions:
Animations can make interactive elements, like buttons or menus, feel more engaging and responsive to user actions, improving the overall user experience.
Best Practices for Using Animations
While animations can greatly enhance user experience, it’s important to use them wisely. Here are some best practices to keep in mind:
Keep It Simple:
Overuse of animations can overwhelm users. Aim for simplicity and coherence in your design.
Performance Considerations:
Animations can be resource-intensive. Optimize your animations to ensure they do not negatively impact the performance of your web application.
Consistency:
Maintain consistency in your animation styles. Consistent animations help create a unified and professional look for your web application.
Common Problems and Solutions
While adding animations to your web application, you might encounter some common issues. Here are a few and how to solve them:
Animations Are Jumpy or Laggy:
Check for performance bottlenecks in your code. Optimizing the use of hardware acceleration, such as using `transform` and `opacity` properties, can help.
Animations Not Triggering:
Ensure that the state change required to trigger the animation is occurring correctly. Double-check your JavaScript events or CSS states.
Conflicting Transitions:
Sometimes multiple animations might conflict. Debug by isolating each animation to identify conflicts and manage their interplay effectively.
Frequently Asked Questions (FAQ)
Can I create animations without using JavaScript?
Yes, you can create animations purely using CSS transitions and CSS keyframes.
What is the difference between CSS transitions and CSS animations?
CSS transitions change property values smoothly over a specified duration when a state change occurs. CSS animations offer more control and use keyframes to define intermediate steps.
How do I optimize animations for performance?
Use hardware-accelerated properties like `transform` and `opacity` for smoother animations.
Can animations be both interactive and responsive?
Yes, combining JavaScript and CSS can create interactive animations that respond to user actions and device changes.
What are some popular libraries for JavaScript animations?
Popular libraries include Anime.js, GreenSock (GSAP), and Velocity.js.
Should I always use JavaScript for complex animations?
Not necessarily. While JavaScript offers enhanced control, CSS keyframes can achieve many complex animations without JavaScript.
Adding animations using JavaScript and CSS transitions can elevate the user experience and make your web applications more engaging. With the examples and best practices discussed, you’re now equipped to enhance your projects with dynamic and smooth animations.
Shop more on Amazon