JavaScript and SVG: Creating Interactive Graphics
Published June 23, 2024 at 3:39 pm
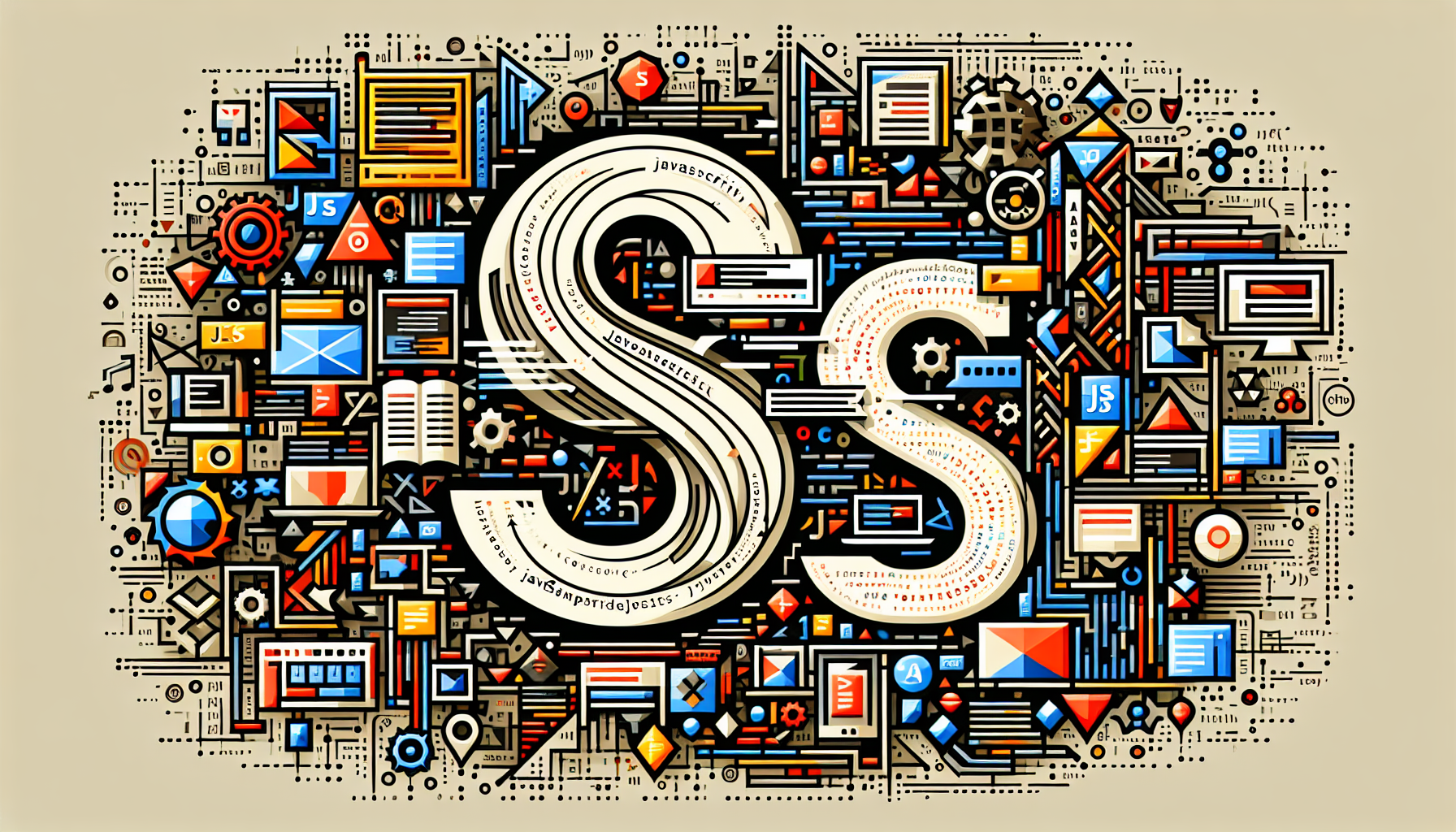
Introduction to JavaScript and SVG
JavaScript and SVG are two technologies that developers use to create interactive web graphics.
SVG stands for Scalable Vector Graphics, a language for describing 2D graphics in XML.
JavaScript is a scripting language that provides dynamic and interactive features on websites.
Combining JavaScript with SVG allows for dynamic manipulation of graphic elements.
TLDR: How to Create Interactive SVG Graphics with JavaScript
To make SVG graphics interactive using JavaScript, you first need to create an SVG element and then manipulate it using JavaScript.
// Step 1: Create an SVG element
const svgElement = document.createElementNS("http://www.w3.org/2000/svg", "svg");
svgElement.setAttribute("width", "500");
svgElement.setAttribute("height", "500");
document.body.appendChild(svgElement);
// Step 2: Create a circle within the SVG
const circle = document.createElementNS("http://www.w3.org/2000/svg", "circle");
circle.setAttribute("cx", "250");
circle.setAttribute("cy", "250");
circle.setAttribute("r", "50");
circle.setAttribute("fill", "blue");
svgElement.appendChild(circle);
// Step 3: Add an event listener to the circle
circle.addEventListener("click", function() {
circle.setAttribute("fill", "red");
});
This snippet demonstrates creating an SVG circle that changes color when clicked.
Next, let’s dive into more details and examples.
Creating and Manipulating SVG Elements
SVG elements are created in HTML using the
Inside the
JavaScript can dynamically manipulate these elements using methods like
For instance, to create a rectangle:
// Create an SVG element
const svgElement = document.createElementNS("http://www.w3.org/2000/svg", "svg");
svgElement.setAttribute("width", "500");
svgElement.setAttribute("height", "500");
document.body.appendChild(svgElement);
// Create a rectangle inside the SVG
const rectangle = document.createElementNS("http://www.w3.org/2000/svg", "rect");
rectangle.setAttribute("x", "50");
rectangle.setAttribute("y", "50");
rectangle.setAttribute("width", "200");
rectangle.setAttribute("height", "100");
rectangle.setAttribute("fill", "green");
svgElement.appendChild(rectangle);
// Add event listener to change rectangle color on mouseover
rectangle.addEventListener("mouseover", function() {
rectangle.setAttribute("fill", "orange");
});
rectangle.addEventListener("mouseout", function() {
rectangle.setAttribute("fill", "green");
});
This snippet creates a green rectangle that turns orange on mouseover and reverts to green on mouseout.
Animating SVG Elements with JavaScript
Animations bring SVG elements to life and can be achieved using JavaScript.
The
For more complex animations, JavaScript libraries like GreenSock (GSAP) can be used.
Here’s an example using CSS transitions and JavaScript for a smooth animation:
// Create an SVG element
const svgElement = document.createElementNS("http://www.w3.org/2000/svg", "svg");
svgElement.setAttribute("width", "500");
svgElement.setAttribute("height", "500");
document.body.appendChild(svgElement);
// Create a circle inside the SVG
const circle = document.createElementNS("http://www.w3.org/2000/svg", "circle");
circle.setAttribute("cx", "250");
circle.setAttribute("cy", "250");
circle.setAttribute("r", "50");
circle.setAttribute("fill", "blue");
circle.style.transition = "all 0.5s ease";
svgElement.appendChild(circle);
// Add event listener to animate the circle
circle.addEventListener("click", function() {
circle.setAttribute("cx", Math.random() * 500);
circle.setAttribute("cy", Math.random() * 500);
});
This code moves the circle to a random position within the SVG canvas when clicked.
Advantages and Disadvantages of Using JavaScript and SVG
Advantages
- SVG is resolution-independent and scales without losing quality.
- JavaScript provides real-time interactivity.
- SVG integrates seamlessly with HTML and CSS.
- Dynamic manipulation of graphics through JavaScript.
Disadvantages
- SVG performance can suffer with very complex graphics.
- JavaScript code can become complex for intricate animations.
- Learning curve for mastering both SVG and JavaScript together.
JavaScript Libraries for SVG Manipulation
Various JavaScript libraries make SVG manipulation easier and more efficient.
One popular library is D3.js, which focuses on data-driven documents.
Another is Snap.svg, which provides simple and powerful methods for working with SVG.
Here is an example using Snap.svg to create and animate an SVG element:
const s = Snap(500, 500); // Create a Snap.svg canvas
// Create a circle
const circle = s.circle(100, 100, 50);
circle.attr({
fill: "#bada55",
stroke: "#000",
strokeWidth: 5
});
// Animate the circle
circle.animate({ cx: 300, cy: 300 }, 1000);
This code animates a circle from one position to another using Snap.svg.
Frequently Asked Questions (FAQs)
What are the benefits of using SVG over other image formats?
SVG is vector-based, meaning it scales without losing quality, and it’s also XML-based, which allows for easier manipulation.
Can SVG be styled with CSS?
Yes, SVG elements can be styled using CSS, just like HTML elements.
How do I make an SVG element draggable?
You can make an SVG element draggable by adding an event listener for “mousedown”, “mousemove”, and “mouseup” events.
Can I use SVG animations for complex interactive applications?
Yes, but for complex animations, using JavaScript libraries like GreenSock or Snap.svg might be beneficial.
Is it possible to create interactive charts and graphs using SVG and JavaScript?
Absolutely, using libraries like D3.js, you can create data-driven interactive charts and graphs.
Adding Interactivity to SVG with Event Listeners
Event listeners in JavaScript are used to add interactivity to SVG elements.
Common events include click, mouseover, and mouseout.
Here’s an example of adding click interactivity to an SVG rectangle:
// Create an SVG element
const svgElement = document.createElementNS("http://www.w3.org/2000/svg", "svg");
svgElement.setAttribute("width", "600");
svgElement.setAttribute("height", "400");
document.body.appendChild(svgElement);
// Create a rectangle inside the SVG
const rectangle = document.createElementNS("http://www.w3.org/2000/svg", "rect");
rectangle.setAttribute("x", "50");
rectangle.setAttribute("y", "50");
rectangle.setAttribute("width", "100");
rectangle.setAttribute("height", "50");
rectangle.setAttribute("fill", "blue");
svgElement.appendChild(rectangle);
// Add click event listener to the rectangle
rectangle.addEventListener("click", function() {
const currentColor = rectangle.getAttribute("fill");
rectangle.setAttribute("fill", currentColor === "blue" ? "green" : "blue");
});
This code snippet demonstrates toggling the color of a rectangle between blue and green when clicked.
Animating SVG Paths with JavaScript
Animating paths in SVG can create complex and intricate animations.
JavaScript can dynamically alter the ‘d’ attribute of paths for animation effects.
Here is an example of animating a path with JavaScript:
// Create an SVG element
const svgElement = document.createElementNS("http://www.w3.org/2000/svg", "svg");
svgElement.setAttribute("width", "600");
svgElement.setAttribute("height", "400");
document.body.appendChild(svgElement);
// Create a path inside the SVG
const path = document.createElementNS("http://www.w3.org/2000/svg", "path");
path.setAttribute("d", "M10 10 L90 90");
path.setAttribute("stroke", "black");
path.setAttribute("stroke-width", "2");
svgElement.appendChild(path);
// Animate the path
let start = null;
function animate(timestamp) {
if (!start) start = timestamp;
const progress = timestamp - start;
const newPosition = 10 + Math.min(90, 0.1 * progress);
path.setAttribute("d", `M10 10 L${newPosition} 90`);
if (newPosition < 90) {
requestAnimationFrame(animate);
}
}
requestAnimationFrame(animate);
This script animates the line drawing of the path from the starting to the ending point.
Understanding Performance Considerations
When combining JavaScript and SVG, performance is crucial.
Complex animations can slow down rendering and impact user experience.
Optimization Techniques
- Minimize the number of SVG elements being animated simultaneously.
- Optimize the SVG structure and reduce unnecessary elements.
- Use requestAnimationFrame for smooth and efficient animations.
- Leverage JavaScript libraries designed for performance.
Creating Responsive SVG Graphics
SVG elements can be made responsive with viewBox and preserveAspectRatio attributes.
These attributes ensure SVG scales up or down based on screen size.
Here's an example of making an SVG element responsive:
// Create an SVG element with viewBox and preserveAspectRatio
const svgElement = document.createElementNS("http://www.w3.org/2000/svg", "svg");
svgElement.setAttribute("viewBox", "0 0 600 400");
svgElement.setAttribute("preserveAspectRatio", "xMidYMid meet");
svgElement.setAttribute("width", "100%");
svgElement.setAttribute("height", "100%");
document.body.appendChild(svgElement);
// Create a circle inside the SVG
const circle = document.createElementNS("http://www.w3.org/2000/svg", "circle");
circle.setAttribute("cx", "300");
circle.setAttribute("cy", "200");
circle.setAttribute("r", "50");
circle.setAttribute("fill", "red");
svgElement.appendChild(circle);
This code adjusts the circle within the SVG to scale with the browser window size.
Handling User Input with Forms Inside SVG
SVG can include forms like input elements to collect user data.
JavaScript handles form input events for dynamic interaction.
Here’s an example of adding an input box inside an SVG element:
// Create an SVG element
const svgElement = document.createElementNS("http://www.w3.org/2000/svg", "svg");
svgElement.setAttribute("width", "600");
svgElement.setAttribute("height", "400");
document.body.appendChild(svgElement);
// Create a foreignObject to include HTML inside SVG
const foreignObject = document.createElementNS("http://www.w3.org/2000/svg", "foreignObject");
foreignObject.setAttribute("x", "10");
foreignObject.setAttribute("y", "10");
foreignObject.setAttribute("width", "200");
foreignObject.setAttribute("height", "80");
svgElement.appendChild(foreignObject);
// Create an input element inside the foreignObject
const input = document.createElement("input");
input.setAttribute("type", "text");
input.setAttribute("placeholder", "Type here...");
foreignObject.appendChild(input);
// Add event listener to handle input changes
input.addEventListener("input", function() {
console.log("User input:", input.value);
});
This example demonstrates embedding an input field within an SVG using a foreignObject.
Enhancing SVG Graphics with Filters and Gradients
Filters and gradients can enhance the visual appeal of SVG elements.
JavaScript can dynamically apply and change these styles.
Here is an example of using a gradient fill for a rectangle:
// Create an SVG element
const svgElement = document.createElementNS("http://www.w3.org/2000/svg", "svg");
svgElement.setAttribute("width", "600");
svgElement.setAttribute("height", "400");
document.body.appendChild(svgElement);
// Define a linear gradient
const defs = document.createElementNS("http://www.w3.org/2000/svg", "defs");
const linearGradient = document.createElementNS("http://www.w3.org/2000/svg", "linearGradient");
linearGradient.setAttribute("id", "gradient1");
linearGradient.setAttribute("x1", "0%");
linearGradient.setAttribute("y1", "0%");
linearGradient.setAttribute("x2", "100%");
linearGradient.setAttribute("y2", "100%");
defs.appendChild(linearGradient);
const stop1 = document.createElementNS("http://www.w3.org/2000/svg", "stop");
stop1.setAttribute("offset", "0%");
stop1.setAttribute("stop-color", "blue");
linearGradient.appendChild(stop1);
const stop2 = document.createElementNS("http://www.w3.org/2000/svg", "stop");
stop2.setAttribute("offset", "100%");
stop2.setAttribute("stop-color", "red");
linearGradient.appendChild(stop2);
svgElement.appendChild(defs);
// Create a rectangle using the gradient
const rectangle = document.createElementNS("http://www.w3.org/2000/svg", "rect");
rectangle.setAttribute("x", "50");
rectangle.setAttribute("y", "50");
rectangle.setAttribute("width", "200");
rectangle.setAttribute("height", "100");
rectangle.setAttribute("fill", "url(#gradient1)");
svgElement.appendChild(rectangle);
This code snippet creates a rectangle with a dynamic linear gradient fill.
Advanced Techniques with JavaScript and SVG
Combining JavaScript with SVG for advanced graphics requires best practices.
Techniques include responsive design, performance optimization, and enhanced interactivity.
Key Techniques
- Use SVG's built-in attributes like viewBox for responsive design.
- Optimize animations with requestAnimationFrame and efficient loops.
- Incorporate third-party libraries like GSAP for advanced animations.
- Leverage browser developer tools to debug and fine-tune your SVG and JavaScript code.
Frequently Asked Questions (FAQs)
What advantages do SVG graphics offer over traditional image formats?
SVG graphics scale without resolution loss and integrate easily with HTML, CSS, and JavaScript.
How can I style SVG elements with CSS?
SVG elements can be styled using CSS just like HTML elements, allowing for consistent styling across your website.
Is it possible to embed HTML elements within an SVG?
Yes, using the
How can I create complex animations for SVG elements?
For complex animations, libraries like GreenSock (GSAP) and Snap.svg are highly effective.
Can I use SVG and JavaScript to create interactive data visualizations?
Absolutely. Libraries like D3.js allow you to create interactive and data-driven visualizations using SVG and JavaScript.
Shop more on Amazon