JavaScript and the History API
Published June 28, 2024 at 6:09 pm
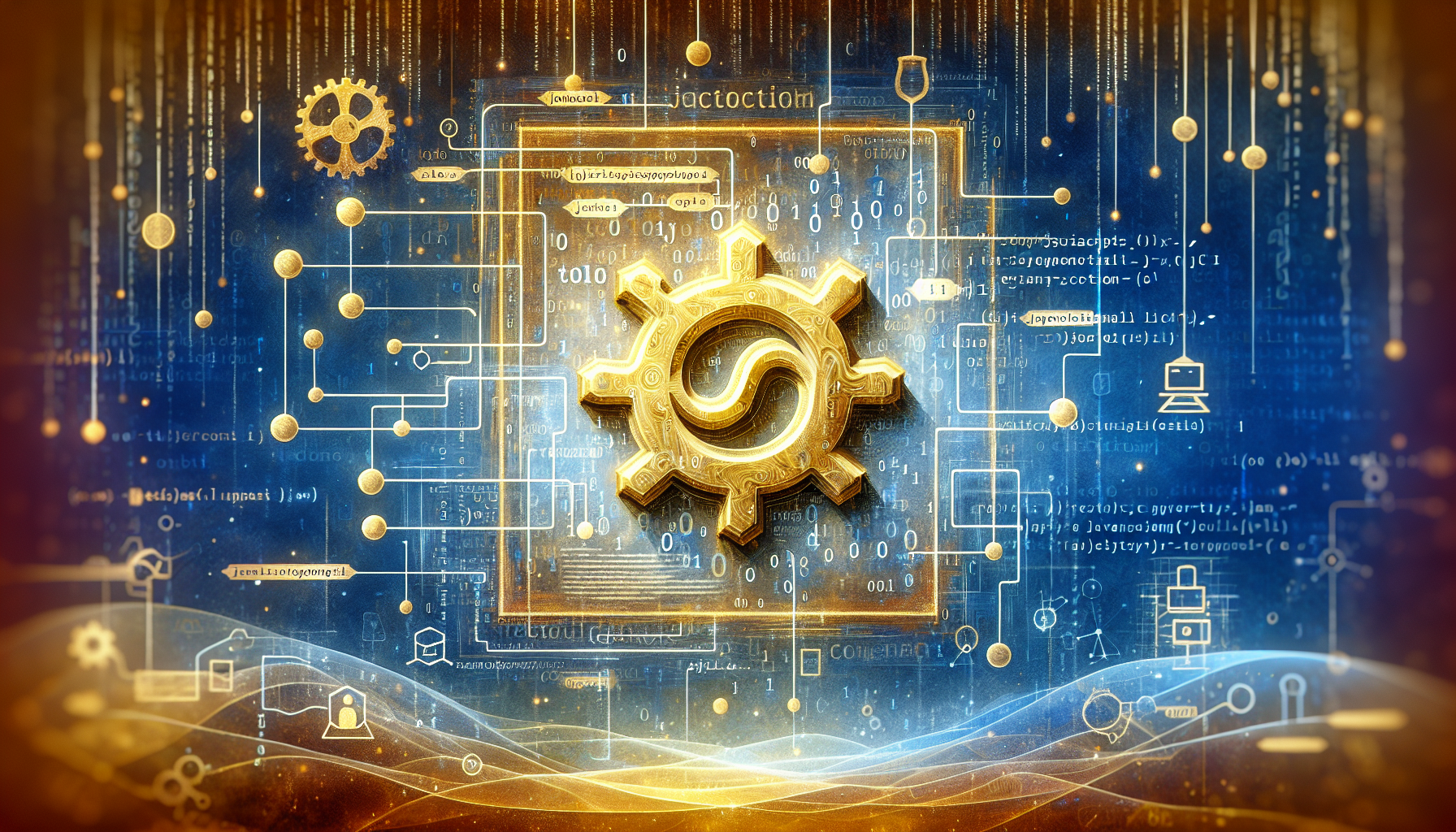
Understanding the History API in JavaScript
The History API is an essential tool in modern JavaScript development.
It allows web developers to manage the browser history directly from JavaScript.
This can create a smoother and more dynamic user experience.
With the History API, developers can manipulate the browser history for single-page applications.
This avoids the need for the page to reload.
TL;DR: How Do I Use the History API in JavaScript?
To manipulate the browser history using the History API in JavaScript, use the methods history.pushState()
, history.replaceState()
, and history.back()
.
For example:
// Add a new state to the history
history.pushState({ id: 1 }, 'Page 1', '/page1');
// Replace the current state
history.replaceState({ id: 2 }, 'Page 2', '/page2');
// Go back to the previous state
history.back();
What Is the History API?
The History API is part of the HTML5 specification.
It allows developers to interact with the browser’s session history.
Session history includes the URLs the user has visited in the current tab.
With the History API, developers can add, change, and remove history entries.
Methods of the History API
There are several key methods of the History API.
These include pushState()
, replaceState()
, and back()
.
pushState()
adds a new entry to the browser’s session history stack.
This method takes three parameters – a state object, a title, and a URL.
Example:
history.pushState({ id: 1 }, 'Page 1', '/page1');
This adds a new state to the history, meaning users can navigate forward and back.
replaceState()
modifies the current history entry.
It takes the same parameters as pushState()
, but it does not create a new history entry.
Example:
history.replaceState({ id: 2 }, 'Page 2', '/page2');
back()
moves back one entry in the history.
Example:
history.back();
Why Use the History API?
The History API is incredibly useful in single-page applications (SPAs).
SPAs load content dynamically without requiring page reloads.
Using the History API in SPAs improves user experience.
It allows the URL to be updated as the user navigates through the app.
This maintains usability and navigational flow.
Pros and Cons of the History API
Pros
- Improves user experience in single-page applications.
- Provides a way to manage state changes without reloading the page.
- Keeps the URL updated and relevant, aiding usability.
Cons
- Requires careful handling to manage state effectively.
- Can lead to complex code structure if not managed well.
- Browser support needs to be checked for compatibility.
Practical Examples
Here are more practical examples to demonstrate how you might use the History API in a project.
Adding and Replacing State
First, let’s add a new state to the history stack.
history.pushState({ page: 1 }, 'Title 1', '?page=1');
This sets the URL to ‘?page=1’ without reloading the page.
Next, replace the current state instead of adding a new one.
history.replaceState({ page: 2 }, 'Title 2', '?page=2');
This changes the URL to ‘?page=2’ without adding another history entry.
Handling Popstate Events
To handle state changes, you need to listen for ‘popstate’ events.
These events are triggered by actions like clicking the browser’s forward or back buttons.
Add an ‘popstate’ event listener to handle these events.
window.addEventListener('popstate', function(event) {
console.log('State:', event.state);
});
Dynamic User Interfaces
You can use the History API to create dynamic user interfaces.
For instance, you might change the content displayed based on the current state.
Update the content when a ‘popstate’ event occurs.
window.addEventListener('popstate', function(event) {
if (event.state && event.state.page) {
var content = document.querySelector('.content');
content.textContent = 'You are viewing page ' + event.state.page;
}
});
Common Issues and How to Fix Them
State Not Being Updated
If the state is not being updated correctly, check your pushState()
and replaceState()
calls.
Ensure you are passing the correct parameters.
Ensure that the browser supports the History API.
Handling Initial State
When your page loads, there might not be an initial state.
To handle this, you might set an initial state when the page loads.
Example:
if (!history.state) {
history.replaceState({ page: 1 }, 'Title 1', '?page=1');
}
Browser Compatibility
Most modern browsers support the History API.
However, there could be issues with older browsers or specific versions.
Check compatibility and include fallbacks if necessary.
Frequently Asked Questions
What are the main methods of the History API?
The main methods are pushState()
, replaceState()
, and back()
.
What is the purpose of the History API?
The purpose is to allow developers to manage the browser history.
This helps create a smoother user experience in web applications.
How does pushState()
work?
It adds a new entry to the history stack.
This allows users to navigate back and forth through application states.
How does replaceState()
work?
It modifies the current history entry without adding a new one.
Why is the History API useful for single-page applications?
It allows changing the URL without reloading the page.
This maintains a seamless user experience.
What are some common issues with the History API?
Common issues include improper state updates, handling of initial state, and browser compatibility.
Ensure correct parameter usage and check browser support to address these issues.
Exploring the History API with Examples
Diving deeper into practical uses of the History API, let’s explore some more complex scenarios.
Using State Objects Effectively
One of the powerful features of the History API is its ability to store state objects.
These objects can store additional information about the state.
For example, you could store the user’s scroll position.
// Save the current scroll position
var stateObj = { scrollY: window.scrollY };
history.pushState(stateObj, 'Page with scroll position', '?page=scroll');
This way, when the user navigates back, you can restore the scroll position.
Restoring State on Popstate
Restoring state when the user navigates back or forward is crucial for a good user experience.
Using the ‘popstate’ event, you can restore the saved state.
window.addEventListener('popstate', function(event) {
if (event.state && event.state.scrollY) {
window.scrollTo(0, event.state.scrollY);
}
});
This ensures the user ends up at the same scroll position they left off.
Advanced State Management
In more complex applications, you might want to store other forms of state.
You could store form data, user preferences, or application-specific details.
// Save user preferences
var preferences = { theme: 'dark', fontSize: 16 };
history.pushState(preferences, 'Preferences Set', '?page=prefs');
This keeps user preferences intact across navigation.
Handling Complex Navigation Flows
In applications with complex navigation flows, careful management of state is essential.
Consider a multi-step form where you save the user’s progress.
// Save form progress
var formData = { step: 2, data: { name: 'John', email: 'john@example.com' } };
history.pushState(formData, 'Step 2', '?page=form-step2');
Restoring the form state on navigation is then straightforward.
window.addEventListener('popstate', function(event) {
if (event.state && event.state.step) {
// Restore form data based on state
var form = document.querySelector('#form');
form.querySelector('input[name="name"]').value = event.state.data.name;
form.querySelector('input[name="email"]').value = event.state.data.email;
}
});
This approach ensures the user doesn’t lose their place in the form.
Common Pitfalls and Overcoming Them
Implementing the History API isn’t without challenges.
Here are some pitfalls and tips on overcoming them.
Managing Large States
Storing too much data in the state object can lead to performance issues.
State objects should be kept small to avoid slowing down the browser.
Ensuring Backward Compatibility
Ensure your application works smoothly in browsers that support the History API.
For older browsers, implement graceful degradation or fallbacks.
Handling History Entry Limits
Browsers have limits on the number of history entries they can store.
Avoid excessive state changes to prevent hitting these limits.
Enhancing User Experience with History API
Using the History API can greatly enhance user experience.
Management of state transitions helps create smoother navigation flows.
Synchronizing URL with State
Synchronizing the URL with application state is crucial for usability.
This ensures users can bookmark, share, or reload the URL without losing context.
Creating More Responsive Interfaces
Interfaces that respond smoothly to user actions feel more intuitive.
The History API allows for seamless state updates in dynamic applications.
Integrating with Other Technologies
The History API works well with various web technologies.
Here are a few integrations you might consider.
Using with AJAX
AJAX and the History API together create a dynamic user experience.
Load new content dynamically and update the URL without reloading the page.
// Fetch new content via AJAX
fetch('/new-content')
.then(response => response.text())
.then(data => {
document.querySelector('.content').innerHTML = data;
// Update history state
history.pushState({ page: 'newContent' }, 'New Content', '/new-content');
});
Integrating with Front-End Frameworks
Many front-end frameworks, like React and Vue, have built-in support for the History API.
These frameworks provide abstractions for handling state and URL synchronization.
Integrate with these frameworks to streamline your application’s state management.
Supporting SEO
Single-page applications benefit from History API for better SEO.
Search engines can crawl different states of your application effectively.
Ensure your application uses unique URLs for different content to improve searchability.
Additional Tips and Best Practices
Here are some additional tips for effectively using the History API.
Working with Browser Compatibility
Always test your application across different browsers and devices.
Ensure consistent behavior and implement fallbacks where necessary.
Optimizing State Management
Keep state objects efficient and avoid storing excessive data.
This improves performance and reduces memory usage.
Maintaining URL Consistency
Always keep the URL in sync with the user’s current view or state.
This aids navigation and enhances the user experience.
Frequently Asked Questions
What are the main methods of the History API?
The main methods are pushState()
, replaceState()
, and back()
.
What is the purpose of the History API?
The purpose is to allow developers to manage the browser history.
This helps create a smoother user experience in web applications.
How does pushState()
work?
It adds a new entry to the history stack.
This allows users to navigate back and forth through application states.
How does replaceState()
work?
It modifies the current history entry without adding a new one.
Why is the History API useful for single-page applications?
It allows changing the URL without reloading the page.
This maintains a seamless user experience.
What are some common issues with the History API?
Common issues include improper state updates, handling of initial state, and browser compatibility.
Ensure correct parameter usage and check browser support to address these issues.
Shop more on AmazonTable of Contents
- Understanding the History API in JavaScript
- What Is the History API?
- Methods of the History API
- Why Use the History API?
- Pros and Cons of the History API
- Practical Examples
- Common Issues and How to Fix Them
- Frequently Asked Questions
- Exploring the History API with Examples
- Common Pitfalls and Overcoming Them
- Enhancing User Experience with History API
- Integrating with Other Technologies
- Additional Tips and Best Practices
- Frequently Asked Questions