JavaScript Best Practices for Beginners
Published June 25, 2024 at 7:14 pm
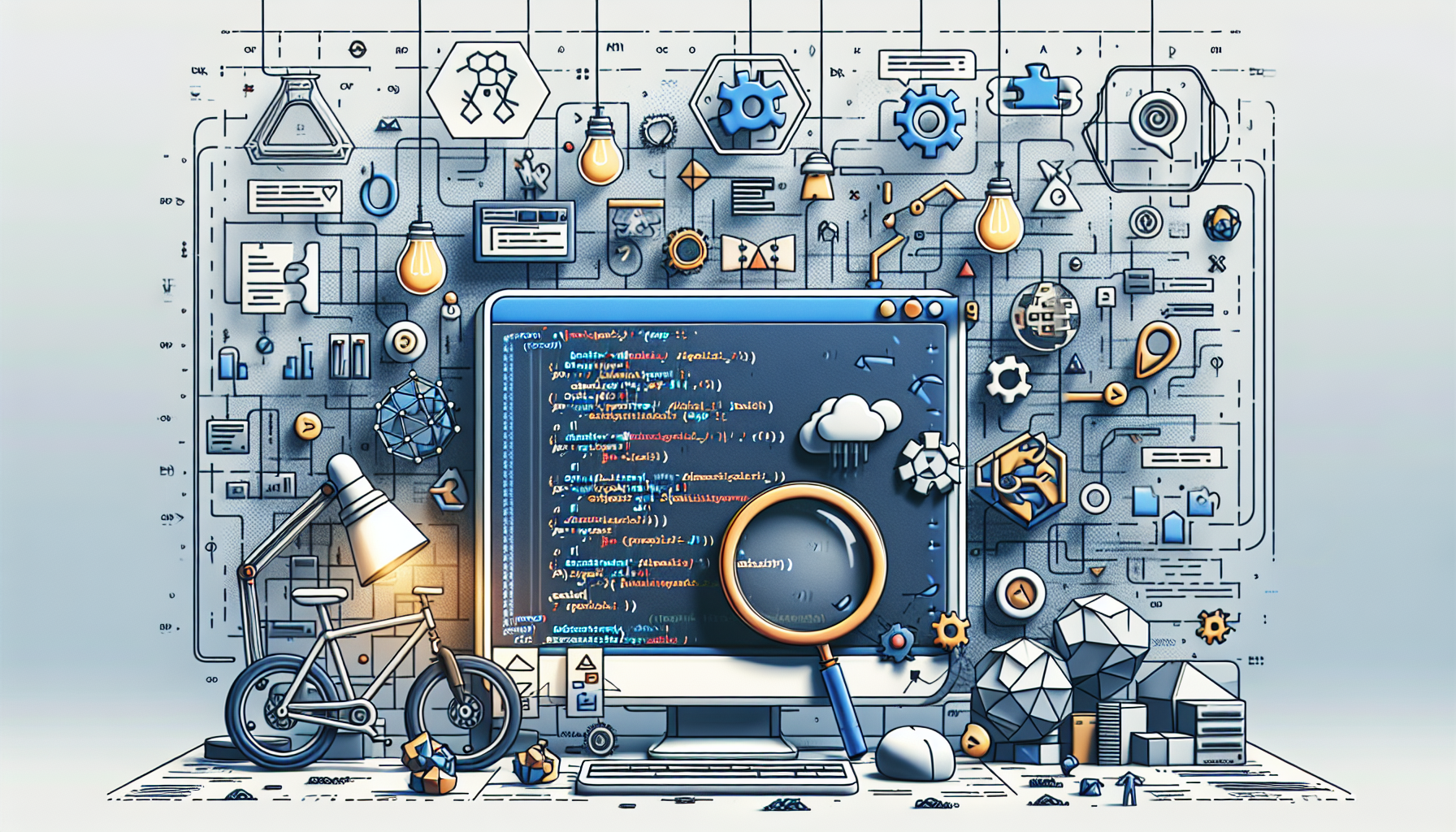
Introduction to JavaScript Best Practices
JavaScript is an essential skill for any web developer, especially beginners. Understanding best practices can significantly improve code quality and maintainability.
**TLDR: Follow these best practices to write better JavaScript code. Use meaningful variable names, avoid global variables, use strict mode, break down complex functions, and adhere to coding standards. Here’s a quick example:**
“`javascript
“use strict”;
// Avoid global variables
(function() {
// Use meaningful names
const itemList = [“item1”, “item2”, “item3”];
// Break down complex functions
itemList.forEach(function(item) {
console.log(item);
});
})();
“`
Adopt Meaningful Variable Names
Use clear and meaningful names for variables and functions. This improves readability.
Avoid single-letter variables unless in loops or very short scopes.
Good naming reduces the need for comments.
Minimize the Use of Global Variables
Global variables can lead to code conflicts and bugs. Keep the scope local when possible.
Encapsulate your code in functions or immediately invoked function expressions (IIFE).
“`javascript
(function() {
const localVariable = “Hello World”;
console.log(localVariable);
})();
“`
Enable Strict Mode
Strict mode in JavaScript helps catch common errors and bugs by enforcing stricter parsing and error handling.
Activate strict mode at the beginning of your scripts or functions with `use strict`.
“`javascript
“use strict”;
function sampleFunction() {
// Your code here
}
“`
Keep Functions Short and Focused
Avoid writing large, monolithic functions. Break them down into smaller, reusable pieces.
This makes your code more modular and easier to maintain.
“`javascript
function calculateTotalPrice(price, tax) {
return price + tax;
}
function displayTotal() {
const totalPrice = calculateTotalPrice(100, 10);
console.log(`Total Price: ${totalPrice}`);
}
displayTotal();
“`
Adhere to Coding Standards
Use a consistent coding style to make your code readable by others. Stick to popular style guides like Airbnb or Google.
Keep your indentation, spaces, and line breaks uniform throughout the code.
“`javascript
// Airbnb Style Guide Example
const isHappy = true;
if (isHappy) {
console.log(“Code is easier to read and maintain!”);
}
“`
Leverage ES6+ Features
JavaScript ES6 and later versions offer many powerful features. Use them to simplify your code.
Features include let, const, template literals, arrow functions, and destructuring.
“`javascript
const user = {
name: “John”,
age: 30
};
const { name, age } = user;
console.log(`User’s Name: ${name}, Age: ${age}`);
“`
Use Promises for Asynchronous Code
Asynchronous operations are better managed with promises or async/await. It helps in avoiding callback hell.
Promises handle asynchronous operations in a more readable way.
“`javascript
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve(“Data fetched successfully!”);
}, 1000);
});
}
fetchData().then(data => {
console.log(data);
});
“`
Optimize Performance
Efficient code runs faster and performs better. Avoid unnecessary calculations within loops.
Use native methods and libraries to optimize your code.
“`javascript
const array = [1, 2, 3, 4, 5];
const newArray = array.map(item => item * 2);
console.log(newArray);
“`
Perform Regular Code Reviews
Regular code reviews help catch issues early and maintain code quality. Collaborate with team members.
The review process encourages knowledge sharing and fosters learning.
Utilize Version Control
Version control systems like Git help track changes and collaborate with other developers. Use descriptive commit messages.
Regularly commit and push your code to repositories like GitHub or GitLab.
“`bash
git init
git add .
git commit -m “Initial commit”
git push origin main
“`
Establish Error Handling Practices
Robust error handling makes your code more resilient. Use try/catch blocks for error-prone code.
Log errors for debugging to make it easier to identify issues.
“`javascript
try {
// Code that may throw an error
const data = JSON.parse(‘{“key”: “value”}’);
console.log(data);
} catch (error) {
console.error(“Error parsing JSON:”, error);
}
“`
FAQ
What are some common pitfalls to avoid in JavaScript?
Avoid using global variables, neglected error handling, and deep nesting in code logic.
Why use `use strict`?
It enforces stricter parsing and error handling, fixing common mistakes and bugs in the code.
How can I avoid callback hell?
Use Promises or async/await for better readability and handling of asynchronous operations.
What are the benefits of using version control?
Version control helps track changes, collaborate easily, and revert to previous versions if needed.
How to improve code readability?
Use meaningful variable names, consistent coding styles, and break down complex functions into smaller ones.
Introduction to JavaScript Best Practices
JavaScript is an essential skill for any web developer, especially beginners. Understanding best practices can significantly improve code quality and maintainability.
Follow these best practices to write better JavaScript code. Use meaningful variable names, avoid global variables, use strict mode, break down complex functions, and adhere to coding standards. Here’s a quick example:
"use strict";
// Avoid global variables
(function() {
// Use meaningful names
const itemList = ["item1", "item2", "item3"];
// Break down complex functions
itemList.forEach(function(item) {
console.log(item);
});
})();
Adopt Meaningful Variable Names
Use clear and meaningful names for variables and functions. This improves readability.
Avoid single-letter variables unless in loops or very short scopes.
Good naming reduces the need for comments.
Minimize the Use of Global Variables
Global variables can lead to code conflicts and bugs. Keep the scope local when possible.
Encapsulate your code in functions or immediately invoked function expressions (IIFE).
(function() {
const localVariable = "Hello World";
console.log(localVariable);
})();
Enable Strict Mode
Strict mode in JavaScript helps catch common errors and bugs by enforcing stricter parsing and error handling.
Activate strict mode at the beginning of your scripts or functions with `use strict`.
"use strict";
function sampleFunction() {
// Your code here
}
Keep Functions Short and Focused
Avoid writing large, monolithic functions. Break them down into smaller, reusable pieces.
This makes your code more modular and easier to maintain.
function calculateTotalPrice(price, tax) {
return price + tax;
}
function displayTotal() {
const totalPrice = calculateTotalPrice(100, 10);
console.log(`Total Price: ${totalPrice}`);
}
displayTotal();
Adhere to Coding Standards
Use a consistent coding style to make your code readable by others. Stick to popular style guides like Airbnb or Google.
Keep your indentation, spaces, and line breaks uniform throughout the code.
// Airbnb Style Guide Example
const isHappy = true;
if (isHappy) {
console.log("Code is easier to read and maintain!");
}
Leverage ES6+ Features
JavaScript ES6 and later versions offer many powerful features. Use them to simplify your code.
Features include let, const, template literals, arrow functions, and destructuring.
const user = {
name: "John",
age: 30
};
const { name, age } = user;
console.log(`User's Name: ${name}, Age: ${age}`);
Use Promises for Asynchronous Code
Asynchronous operations are better managed with promises or async/await. It helps in avoiding callback hell.
Promises handle asynchronous operations in a more readable way.
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data fetched successfully!");
}, 1000);
});
}
fetchData().then(data => {
console.log(data);
});
Optimize Performance
Efficient code runs faster and performs better. Avoid unnecessary calculations within loops.
Use native methods and libraries to optimize your code.
const array = [1, 2, 3, 4, 5];
const newArray = array.map(item => item * 2);
console.log(newArray);
Perform Regular Code Reviews
Regular code reviews help catch issues early and maintain code quality. Collaborate with team members.
The review process encourages knowledge sharing and fosters learning.
Utilize Version Control
Version control systems like Git help track changes and collaborate with other developers. Use descriptive commit messages.
Regularly commit and push your code to repositories like GitHub or GitLab.
git init
git add .
git commit -m "Initial commit"
git push origin main
Establish Error Handling Practices
Robust error handling makes your code more resilient. Use try/catch blocks for error-prone code.
Log errors for debugging to make it easier to identify issues.
try {
// Code that may throw an error
const data = JSON.parse('{"key": "value"}');
console.log(data);
} catch (error) {
console.error("Error parsing JSON:", error);
}
Frequently Asked Questions (FAQ)
What are some common pitfalls to avoid in JavaScript?
Avoid using global variables, neglected error handling, and deep nesting in code logic.
Why use `use strict`?
It enforces stricter parsing and error handling, fixing common mistakes and bugs in the code.
How can I avoid callback hell?
Use Promises or async/await for better readability and handling of asynchronous operations.
What are the benefits of using version control?
Version control helps track changes, collaborate easily, and revert to previous versions if needed.
How to improve code readability?
Use meaningful variable names, consistent coding styles, and break down complex functions into smaller ones.
Shop more on Amazon