JavaScript Data Types Explained
Published June 5, 2024 at 8:05 pm
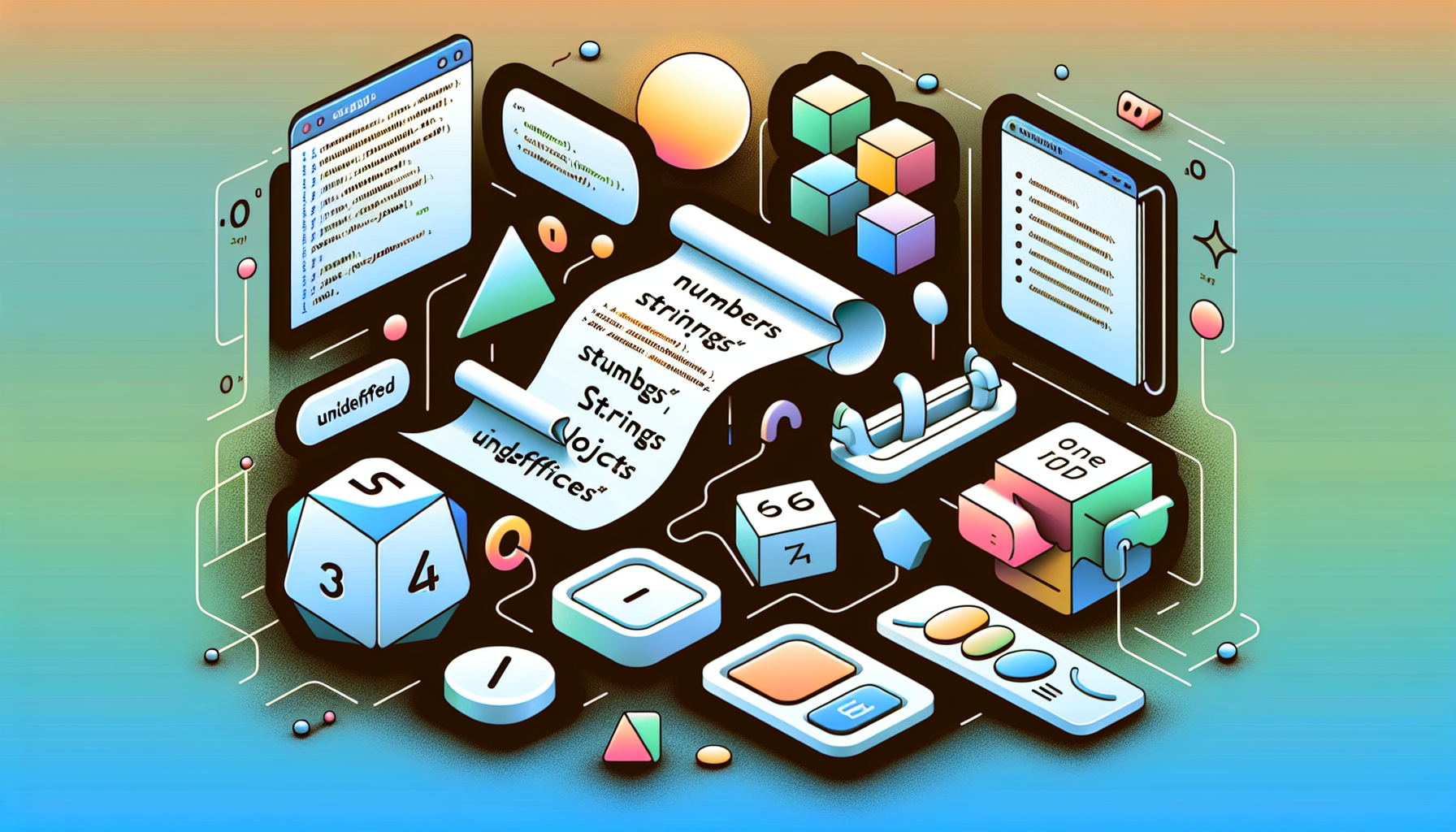
An Overview of JavaScript Data Types
Are you new to JavaScript and struggling to understand its data types? JavaScript simplifies data management by offering a variety of data types, each suited for different scenarios.
Answer: JavaScript has seven primary data types: six primitive types (String, Number, Boolean, Null, Undefined, Symbol) and one complex type (Object).
We’ll break down each type and provide examples to illustrate their use.
Primitive Data Types in JavaScript
Primitive data types are the most basic data types in JavaScript. They include String, Number, Boolean, Null, Undefined, and Symbol.
String
Strings are sequences of characters used for textual data.
They are created by enclosing characters in quotes, either single or double.
// Example of String
var greet = "Hello, World!"; // Using double quotes
var name = 'John Doe'; // Using single quotes
Number
Numbers represent numeric values.
JavaScript handles integers and floating-point numbers as the same type.
// Example of Number
var age = 30; // Integer
var score = 4.5; // Floating-point number
Boolean
Boolean data type can only hold two values: true or false.
They are commonly used in conditional testing.
// Example of Boolean
var isStudent = true;
var hasGraduated = false;
Null
The Null data type represents the intentional absence of any object value.
It indicates a deliberate non-value.
// Example of Null
var result = null;
Undefined
Undefined indicates that a variable has not been assigned a value.
Variables declared but not initialized hold the undefined value.
// Example of Undefined
var unassigned;
console.log(unassigned); // Output: undefined
Symbol
Symbol is a unique and immutable data type.
It is primarily used for private object properties.
// Example of Symbol
var uniqueID = Symbol('id');
console.log(uniqueID); // Output: Symbol(id)
Complex Data Type in JavaScript
Besides the primitive data types, JavaScript has one complex data type: Object.
Object
Objects are collections of key-value pairs.
They can store multiple values in a single variable.
// Example of Object
var user = {
name: 'Alice',
age: 25,
isAdmin: false
};
console.log(user.name); // Output: Alice
TLDR: How to Use JavaScript Data Types
String: Enclose characters in quotes (e.g., var name = “John”;).
Number: Use numeric values, including integers and floats (e.g., var score = 100;).
Boolean: Use true or false values (e.g., var isStudent = true;).
Null: Assign null to indicate a deliberate non-value (e.g., var result = null;).
Undefined: Variables without assignments hold the value undefined (e.g., var x;).
Symbol: Create unique identifiers for object properties (e.g., var id = Symbol(‘id’);).
Object: Store related data with key-value pairs (e.g., var user = {name: “Alice”};).
Understanding Type Coercion
Type coercion refers to the automatic or implicit conversion of values from one data type to another.
This conversion happens when operators or functions expect a specific type.
// Example of Type Coercion
console.log('5' - 2); // Output: 3 (String '5' is converted to Number 5)
console.log('5' + 2); // Output: 52 (Number 2 is converted to String '2')
JavaScript converts data types to fit the context.
Checking Data Types
To check the data type of a variable, we can use the typeof
operator.
// Example of typeof
console.log(typeof 'Hello'); // Output: string
console.log(typeof 42); // Output: number
console.log(typeof true); // Output: boolean
The typeof
operator helps us identify the data type.
Common Issues and How to Fix Them
How do I avoid type coercion errors?
Use strict comparison operators (=== or !==).
Explicitly convert data using functions like Number() or String().
What is the difference between null and undefined?
Null is an intentional absence of any value.
Undefined is a variable that has been declared but not assigned a value.
How can I create a new object?
Use object literals (var user = {};) or constructor functions (var user = new Object();).
Examples and Best Practices
In this section, we will cover best practices for working with data types in JavaScript.
// Using object literals
var person = {
firstName: 'Jane',
lastName: 'Doe',
age: 32
};
// Using constructor function
var person = new Object();
person.firstName = 'Jane';
person.lastName = 'Doe';
person.age = 32;
Using object literals is often preferred for simplicity.
How can I append a property to an existing object?
You can directly add the property using dot notation or bracket notation.
// Using Dot Notation
person.address = '123 Main St';
// Using Bracket Notation
person['address'] = '123 Main St';
Both methods are valid and can be used based on preference or specific needs.
How do I convert other data types to string?
To convert data to a string format, use the String()
function or .toString()
method.
// Using String() function
var age = 25;
var ageAsString = String(age); // '25'
// Using .toString() method
var flag = true;
var flagAsString = flag.toString(); // 'true'
These methods ensure that data is converted to a string type.
Conclusion and Content area 2 of 2
Congratulations on making it through this comprehensive guide on JavaScript data types.
We have covered the basics of primitive and complex data types.
Understanding these concepts is crucial for effective JavaScript programming.
By mastering data types, you lay a solid foundation for building complex applications.
Keep practicing and experimenting with these concepts in your projects.
Happy coding!
Type Conversion in JavaScript
Type conversion allows you to explicitly change the type of a variable to another type.
This process is sometimes necessary for computations and comparisons.
Implicit Type Conversion
Implicit type conversion, also known as coercion, is automatically performed by JavaScript.
// Example of Implicit Type Conversion
console.log('4' * 2); // Output: 8 (String '4' is converted to Number 4)
console.log('5' + true); // Output: '5true' (Boolean true is converted to String 'true')
JavaScript performs implicit conversion based on the context.
Explicit Type Conversion
Explicit conversion is performed using helper functions like Number()
, String()
, and Boolean()
.
// Example of Explicit Type Conversion
var str = '123';
var num = Number(str); // Converts string to number
console.log(num); // Output: 123
var n = 456;
var strNum = String(n); // Converts number to string
console.log(strNum); // Output: '456'
Explicit conversion ensures that data types are correctly transformed.
Arrays in JavaScript
Arrays are ordered collections of values and are one of the key data structures in JavaScript.
They can hold values of different data types and are created using square brackets.
Creating and Initializing Arrays
To create an array, use square brackets and separate values with commas.
// Example of Array
var fruits = ['Apple', 'Banana', 'Cherry'];
console.log(fruits[0]); // Output: Apple
Each value in an array can be accessed using its index.
Common Array Methods
JavaScript provides a variety of methods for array manipulation.
// Example of Array Methods
var fruits = ['Apple', 'Banana', 'Cherry'];
// Adding an item to the end
fruits.push('Orange');
console.log(fruits); // Output: ['Apple', 'Banana', 'Cherry', 'Orange']
// Removing the last item
fruits.pop();
console.log(fruits); // Output: ['Apple', 'Banana', 'Cherry']
// Adding an item to the beginning
fruits.unshift('Strawberry');
console.log(fruits); // Output: ['Strawberry', 'Apple', 'Banana', 'Cherry']
// Removing the first item
fruits.shift();
console.log(fruits); // Output: ['Apple', 'Banana', 'Cherry']
These methods allow for easy and dynamic manipulation of array elements.
Functions and Their Importance
Functions are blocks of reusable code that perform a specific task.
They help break the code into modular, manageable, and reusable components.
Defining Functions
Functions are defined using the function
keyword, followed by a name, parameters in parentheses, and a block of code in curly braces.
// Example of Function
function greet(name) {
return 'Hello, ' + name + '!';
}
console.log(greet('Alice')); // Output: Hello, Alice!
Functions encapsulate code for specific tasks, making them reusable.
Anonymous Functions
Anonymous functions are functions without a name.
They are often used as arguments to other functions, such as event handlers and callbacks.
// Example of Anonymous Function
var sum = function(a, b) {
return a + b;
};
console.log(sum(5, 3)); // Output: 8
Anonymous functions are useful in situations where a function is needed temporarily.
Arrow Functions in JavaScript
Arrow functions provide a concise syntax for writing functions.
They use the =>
syntax and are particularly useful for short functions.
Syntax of Arrow Functions
Arrow functions can be defined without the function
keyword.
// Example of Arrow Function
const add = (x, y) => x + y;
console.log(add(2, 3)); // Output: 5
Arrow functions are especially handy for simple one-liner functions.
Differences Between Arrow Functions and Regular Functions
Arrow functions do not have their own this
context.
This characteristic makes them unique compared to regular functions.
// Example of 'this' in Arrow Function
const person = {
name: 'Jane',
greet: function() {
return () => 'Hello, ' + this.name;
}
};
const greeting = person.greet();
console.log(greeting()); // Output: Hello, Jane
Arrow functions inherit the this
context from their enclosing scope.
Frequently Asked Questions
What is the difference between declaring a variable with let
and var
?
let
is block-scoped and var
is function-scoped.
What are template literals?
Template literals allow for embedded expressions and multi-line strings using backticks (`
).
How do I create a new array from an existing array?
Use array methods like map()
or filter()
to create new arrays.
What is the difference between null
and undefined
?
null
represents the intentional absence of any value, while undefined
indicates a variable has not been assigned a value.
How can I check if an object has a specific property?
Use the hasOwnProperty()
method.
How do I convert a string to a number in JavaScript?
Use functions like Number()
or parseInt()
.
What is a callback function?
A callback function is a function passed as an argument to another function to be executed later.
How can I merge two or more arrays?
Use the concat()
method or the spread operator (...
).
How do I avoid common pitfalls with this
in JavaScript?
Understand the context in which this
is used or use bind, call, apply methods to control this
context.