JavaScript Promises: Handling Async Operations
Published June 28, 2024 at 5:46 pm
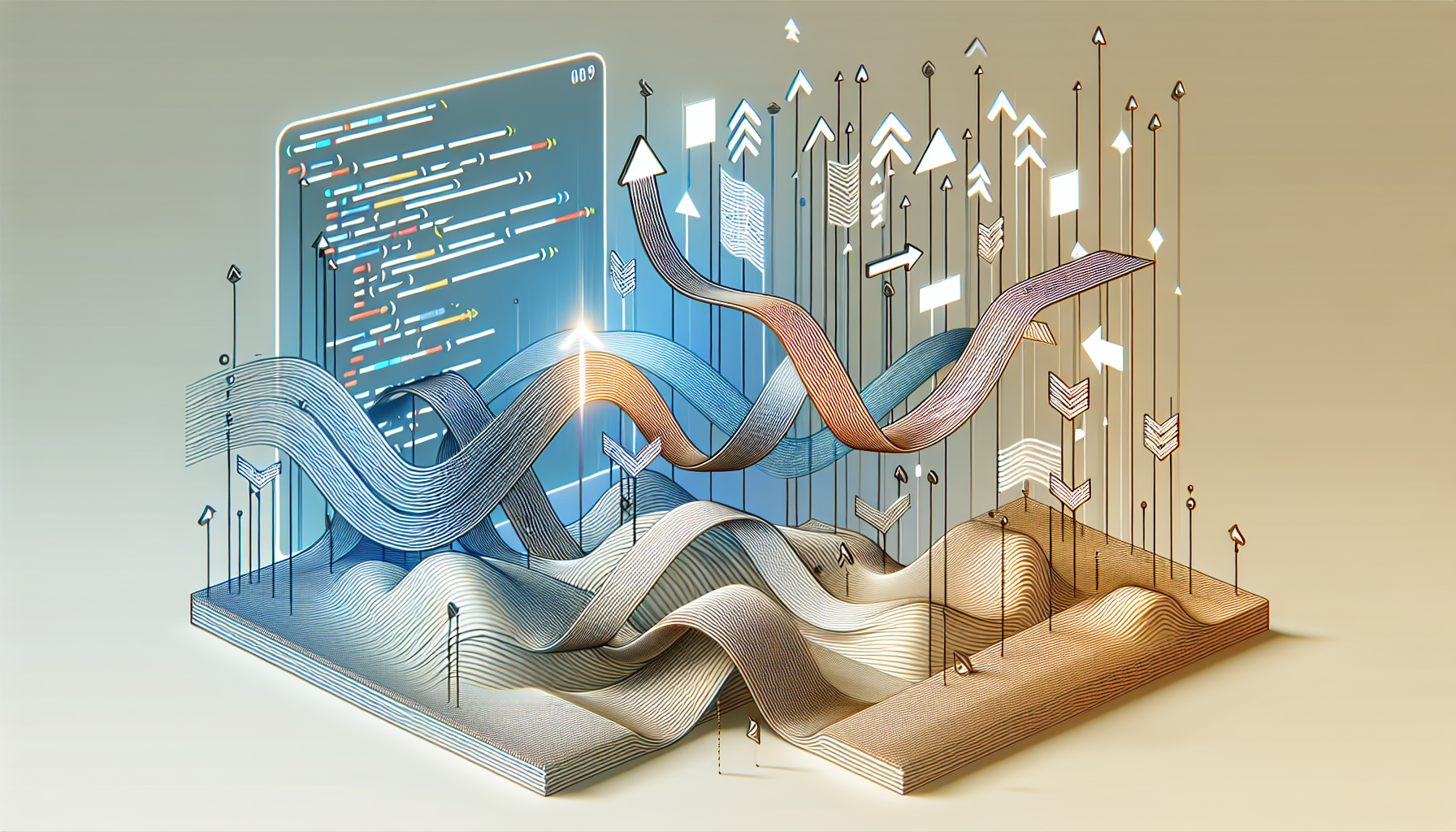
Introduction to JavaScript Promises in Async Operations
JavaScript promises provide a powerful way to handle asynchronous operations.
This can be very useful when dealing with APIs, databases, or any operation that takes time to complete.
Promises improve readability and make the code more manageable.
If you’re used to callback functions, promises offer a cleaner and more intuitive approach.
Understanding promises will help you write more efficient and effective JavaScript code.
TL;DR: How to Use JavaScript Promises for Async Operations:
To handle async operations, create a promise using the new Promise
constructor.
Use the .then()
method to handle resolved promises and .catch()
for errors.
Example:
// Create a new promise
let myPromise = new Promise((resolve, reject) => {
// Simulate an asynchronous operation using setTimeout
setTimeout(() => {
let success = true; // change to false to test reject
if (success) {
resolve('Promise resolved successfully.');
} else {
reject('Promise was rejected.');
}
}, 2000); // 2-second delay
});
// Handle the promise
myPromise.then((message) => {
console.log(message); // logs 'Promise resolved successfully.'
}).catch((error) => {
console.error(error); // logs 'Promise was rejected.'
});
What Are JavaScript Promises?
A JavaScript promise is an object that represents the eventual completion or failure of an asynchronous operation.
It’s a placeholder for a value that will be available in the future.
The promise object has three states: pending, fulfilled, and rejected.
Initially, a promise is in the pending state.
If the asynchronous operation is successful, the promise becomes fulfilled.
If the operation fails, the promise becomes rejected.
Basic Usage of Promises
Creating a promise involves using the new Promise
constructor.
The constructor takes a function as an argument, known as the executor.
The executor function receives two arguments: resolve and reject.
Use resolve to mark the promise as fulfilled and reject to mark it as rejected.
Example:
// Create a new promise that resolves after 1 second
let examplePromise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Promise fulfilled successfully.');
}, 1000);
});
// Handle the promise
examplePromise.then((message) => {
console.log(message); // logs 'Promise fulfilled successfully.' after 1 second
}).catch((error) => {
console.error(error); // logs if promise is rejected
});
Chaining Promises
One of the main advantages of promises is the ability to chain them together.
This means you can perform multiple asynchronous operations in sequence.
The .then()
method returns a new promise, making it possible to chain multiple .then()
calls.
Example:
// Chaining promises
let chainPromise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Step 1 complete.');
}, 1000);
});
chainPromise.then((message) => {
console.log(message); // logs 'Step 1 complete.'
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Step 2 complete.');
}, 1000);
});
}).then((message) => {
console.log(message); // logs 'Step 2 complete.'
return 'Step 3 complete.';
}).then((message) => {
console.log(message); // logs 'Step 3 complete.'
}).catch((error) => {
console.error(error); // handles any rejected promise in the chain
});
Using Async/Await with Promises
Async/await is a modern way to work with promises, making the code cleaner and easier to read.
Functions declared with the async
keyword return a promise.
The await
keyword is used to wait for a promise to resolve.
This approach avoids the need for chaining .then()
calls, simplifying the code.
Example:
// Function to simulate an asynchronous operation
const asyncOperation = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Async/await operation complete.');
}, 2000);
});
};
// Using async/await
const asyncFunction = async () => {
try {
const result = await asyncOperation();
console.log(result); // logs 'Async/await operation complete.'
} catch (error) {
console.error(error); // handles any errors
}
};
asyncFunction();
Common Use Cases for Promises
Promises are widely used in various scenarios involving asynchronous operations.
They are commonly used for API calls, handling user input, and database queries.
Using promises makes the code more predictable and easier to debug.
Handling API Calls
Promises are perfect for handling API calls, which are inherently asynchronous.
Example of using the Fetch API with promises:
// Using fetch API to make an HTTP request
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json())
.then(data => {
console.log(data); // logs the array of posts
})
.catch(error => {
console.error('Error fetching data:', error);
});
Error Handling with Promises
Proper error handling is crucial in asynchronous operations.
Promises provide the .catch()
method to handle errors.
Errors can also be handled using a second argument in .then()
.
Example:
// Example with .catch()
let errorPromise = new Promise((resolve, reject) => {
setTimeout(() => {
reject('Error occurred.');
}, 1000);
});
errorPromise.then((message) => {
console.log(message);
}).catch((error) => {
console.error(error); // logs 'Error occurred.'
});
Pros of Using Promises
Advantages of Promises
- Improves code readability and maintains structure.
- Supports chaining for sequential asynchronous operations.
- Facilitates better error handling with
.catch()
method. - Reduces callback hell, making the code neater.
Cons of Using Promises
Disadvantages of Promises
- Learning curve for beginners can be steep.
- Errors can propagate silently if not handled properly.
- May require refactoring of existing callback-based code.
Frequently Asked Questions (FAQs)
What is the difference between Promises and Callbacks?
Promises are more readable and modular than callbacks.
They support chaining and better error handling.
How do I handle multiple promises at once?
Use Promise.all()
to handle multiple promises in parallel.
It returns a single promise that resolves when all input promises are resolved.
Can I cancel a promise?
JavaScript promises do not natively support cancellation.
Use workarounds like manual checks for cancellation status within the promise.
How do I avoid unhandled promise rejections?
Always use .catch()
or add a rejection handler to your promises.
This ensures that errors are properly handled and not ignored.
What is the purpose of the .finally()
method?
The .finally()
method executes code after a promise is settled, regardless of the outcome.
It is useful for cleanup operations and ensuring consistent behavior.
Using Promises for User Input
JavaScript promises can also be useful for handling user input, such as form submissions.
They can help deal with input validation and asynchronous server-side processing.
Example:
// Simulating an async form submission
const formSubmit = (userData) => {
return new Promise((resolve, reject) => {
setTimeout(() => {
if (userData.username && userData.email) {
resolve('Form submitted successfully.');
} else {
reject('Form submission failed.');
}
}, 1500);
});
};
// Handling form submission using a promise
const userData = { username: 'JohnDoe', email: 'john@example.com' };
formSubmit(userData).then((message) => {
console.log(message); // logs 'Form submitted successfully.'
}).catch((error) => {
console.error(error); // logs 'Form submission failed.'
});
Handling Database Queries
Promises are often used in server-side code to handle database queries.
This is because querying a database is an asynchronous operation.
Example using a mock database query:
// Mock database query function
const dbQuery = (query) => {
return new Promise((resolve, reject) => {
setTimeout(() => {
const dbResults = ['data1', 'data2', 'data3'];
if (dbResults.length > 0) {
resolve(dbResults);
} else {
reject('No data found.');
}
}, 2000);
});
};
// Handling database query result
dbQuery('SELECT * FROM table').then((results) => {
console.log(results); // logs array of data
}).catch((error) => {
console.error(error); // logs 'No data found.'
});
Handling Multiple Promises
When dealing with multiple asynchronous operations, you can use JavaScript’s Promise.all()
method.
This method allows you to wait for all the promises to resolve before proceeding.
Example:
// Simulate multiple asynchronous operations
const asyncTask1 = new Promise((resolve) => setTimeout(() => resolve('Task 1 complete'), 1000));
const asyncTask2 = new Promise((resolve) => setTimeout(() => resolve('Task 2 complete'), 1500));
// Using Promise.all to handle multiple promises
Promise.all([asyncTask1, asyncTask2]).then((results) => {
console.log(results); // logs ['Task 1 complete', 'Task 2 complete']
}).catch((error) => {
console.error(error); // handles any rejection
});
Error Handling with Multiple Promises
It’s important to handle errors when working with multiple promises.
Using Promise.all()
will reject if any promise in the array rejects.
Example:
// Simulate multiple asynchronous operations with one failing
const task1 = new Promise((resolve) => setTimeout(() => resolve('Task 1 complete'), 1000));
const task2 = new Promise((_, reject) => setTimeout(() => reject('Task 2 failed'), 1500));
// Using Promise.all to handle multiple promises
Promise.all([task1, task2]).then((results) => {
console.log(results);
}).catch((error) => {
console.error(error); // logs 'Task 2 failed'
});
Using .finally()
with Promises
The .finally()
method is useful for executing code after a promise is settled.
This happens regardless of whether the promise resolves or rejects.
Example:
// Simulate an asynchronous operation
const exampleOperation = new Promise((resolve, reject) => {
setTimeout(() => {
reject('Operation failed.');
}, 1000);
});
exampleOperation.then((message) => {
console.log(message);
}).catch((error) => {
console.error(error); // logs 'Operation failed.'
}).finally(() => {
console.log('Operation complete.'); // logs regardless of resolve or reject
});
Handling Promises in a Loop
Handling promises in a loop can be challenging, but the Promise.all()
method simplifies it.
Example:
// Simulate multiple asynchronous operations in a loop
const tasks = [1, 2, 3].map((task) => {
return new Promise((resolve) => setTimeout(() => resolve(`Task ${task} complete`), task * 1000));
});
// Handle all promises
Promise.all(tasks).then((results) => {
console.log(results); // logs array of task completion messages
}).catch((error) => {
console.error(error); // handles any rejection
});
Handling Conditional Promises
In some cases, you might need to handle promises based on conditional logic.
This can be achieved using nested promises or conditional statements.
Example:
// Simulate a conditional asynchronous operation
const conditionalOperation = (flag) => {
return new Promise((resolve, reject) => {
if (flag) {
resolve('Condition met.');
} else {
reject('Condition not met.');
}
});
};
// Handling conditional promise
conditionalOperation(true).then((message) => {
console.log(message); // logs 'Condition met.'
}).catch((error) => {
console.error(error); // logs 'Condition not met.'
});
Best Practices for Using Promises
Promises are a powerful tool, but there are best practices to follow for optimal use.
Always handle errors using .catch()
or a second argument in .then()
.
Use Promise.all()
for running multiple promises concurrently.
Leverage async/await
syntax for cleaner and more readable code.
Common Mistakes with Promises
A common mistake is not handling promise rejections properly.
Another mistake is nesting promises unnecessarily, leading to messy code.
Avoiding these mistakes can lead to more efficient and maintainable code.
FAQ
What are the benefits of using promises over callbacks?
Promises offer better readability and maintainability of code.
They enable chaining and structured error handling.
Can I use async/await with promises?
Yes, async/await syntax works seamlessly with promises.
It simplifies asynchronous code and makes it look synchronous.
How do I run multiple promises concurrently?
Use Promise.all()
to run multiple promises concurrently.
It waits for all promises to resolve and returns their results in an array.
How can I handle errors in promises?
Use the .catch()
method to handle errors.
Alternatively, provide a second argument to the .then()
method.
What does the .finally()
method do?
The .finally()
method executes code after a promise is settled.
It runs regardless of whether the promise was resolved or rejected.
Shop more on Amazon