JavaScript: Understanding Hoisting
Published June 30, 2024 at 8:11 pm
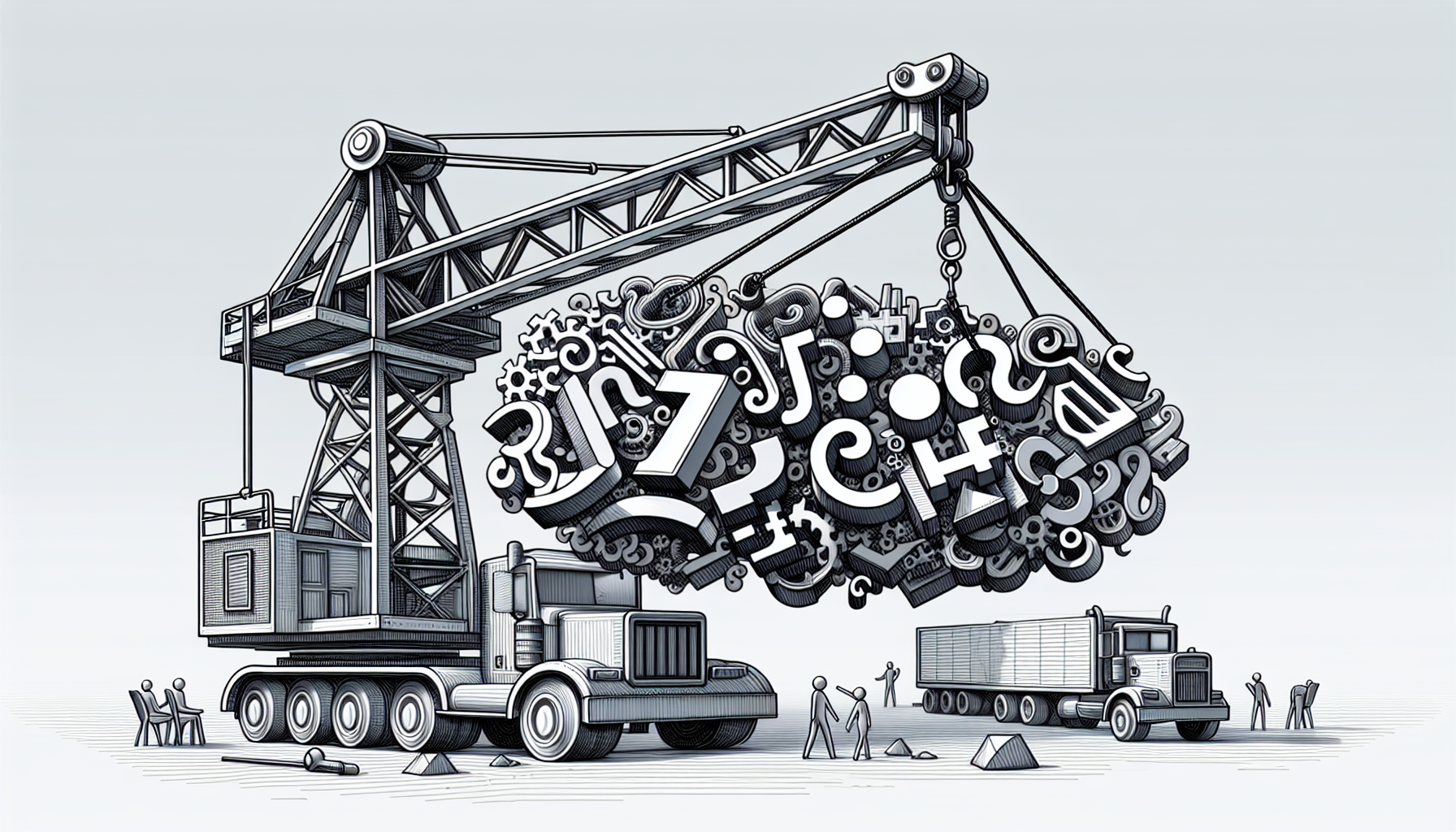
What is Hoisting in JavaScript?
Hoisting is a fundamental concept in JavaScript that developers often encounter.
It is the process where variable and function declarations are moved to the top of their containing scope during the compile phase.
TL;DR: Understanding Hoisting in JavaScript
Hoisting allows you to use functions and variables before they are declared in the code.
// Example of Function Hoisting
hoistedFunction(); // Outputs: "This function has been hoisted."
function hoistedFunction() {
console.log("This function has been hoisted.");
}
// Example of Variable Hoisting
console.log(hoistedVar); // Outputs: undefined
var hoistedVar = "This variable has been hoisted.";
In this example, both the function and the variable are used before they are declared.
This is possible due to hoisting.
How Does Hoisting Work in JavaScript?
To understand hoisting, you need to know that JavaScript has two phases: the creation phase and the execution phase.
During the creation phase, the JavaScript engine allocates memory for variables and functions.
This is where hoisting takes place.
Function declarations are stored entirely in memory during the creation phase.
Variable declarations are stored but initialized as undefined.
This is why you can call a function before it is declared.
However, if you try to access a variable before it is declared, it will return undefined.
Function and Variable Hoisting: A Closer Look
Hoisting behaves differently for function declarations and variable declarations.
For function declarations, the entire function is hoisted.
hoistedFunction(); // Outputs: "This function has been hoisted."
function hoistedFunction() {
console.log("This function has been hoisted.");
}
In this example, the entire function is hoisted to the top of the scope.
This allows you to call the function before it is declared.
For variable declarations, only the declaration is hoisted, not the initialization.
console.log(hoistedVar); // Outputs: undefined
var hoistedVar = "This variable has been hoisted.";
In this example, the variable is hoisted but its value is not assigned until the execution phase.
This is why it returns undefined when accessed before the assignment.
Pros and Cons of Hoisting
Pros
- Allows functions to be called before they are declared, improving code organization.
- Helps avoid reference errors by initializing variables as undefined.
Cons
- Can lead to confusing code behavior if developers are not aware of hoisting.
- May result in unexpected undefined values when accessing variables before initialization.
The Temporal Dead Zone
With the introduction of let and const in ES6, a new concept called the Temporal Dead Zone (TDZ) was introduced.
TDZ refers to the time between entering a scope and the point where a variable is declared.
Accessing a variable during this period results in a ReferenceError.
console.log(hoistedLet); // ReferenceError: Cannot access 'hoistedLet' before initialization
let hoistedLet = "This is a let variable.";
In this example, accessing the let variable before its declaration results in an error.
This is due to the TDZ.
Best Practices to Avoid Issues with Hoisting
Hoisting can lead to unexpected behavior in your code.
Here are some best practices to minimize issues caused by hoisting.
Always declare variables at the top of their scope to avoid confusion.
Use let and const instead of var as they are block-scoped and reduce unexpected behavior related to hoisting.
Declare functions before calling them to make your code more readable and maintainable.
FAQs
What happens when you access a variable before its declaration?
If the variable is declared using var, it will return undefined due to hoisting.
If it is declared using let or const, it will throw a ReferenceError due to the Temporal Dead Zone.
Do function expressions get hoisted?
No, only function declarations are hoisted.
Function expressions are not hoisted.
Can you use hoisting with let and const?
Variables declared with let and const are not hoisted in the same way as var.
They are in the Temporal Dead Zone until their declaration is encountered.
Common Pitfalls of Hoisting
Despite the benefits, hoisting can introduce several pitfalls in your code.
Understanding these pitfalls can help you write more predictable and error-free code.
One common pitfall is the appearance of undefined variables.
This can occur when variables are accessed before their actual initialization in the code.
Another pitfall is assuming functions defined through function expressions are hoisted.
In reality, they are not, which can lead to unexpected behavior.
Variable Hoisting in Different Scopes
Hoisting behaves differently in different scopes.
In the global scope, variables are hoisted to the top of the global execution context.
This means they can be accessed anywhere in the code.
console.log(globalVar); // Outputs: undefined
var globalVar = "This is a global variable.";
In this example, the global variable is hoisted, so accessing it before initialization returns undefined.
In the function scope, variables are hoisted to the top of the function.
This means they can be accessed anywhere within the function.
function exampleFunction() {
console.log(localVar); // Outputs: undefined
var localVar = "This is a local variable.";
}
exampleFunction();
In this example, the local variable is hoisted to the top of the function scope.
This allows it to be accessed anywhere within the function, but before initialization, it returns undefined.
Function Hoisting in Different Scopes
Function hoisting behaves similarly to variable hoisting but with some differences.
In the global scope, function declarations are hoisted to the top of the global execution context.
This means they can be called anywhere in the code.
hoistedFunction(); // Outputs: "This function has been hoisted."
function hoistedFunction() {
console.log("This function has been hoisted.");
}
In this example, the function declaration is hoisted, allowing it to be called before its actual declaration.
In the function scope, function declarations are hoisted to the top of the function.
This means they can be called anywhere within the function.
function exampleFunction() {
hoistedFunction();
function hoistedFunction() {
console.log("This function has been hoisted within the function scope.");
}
}
exampleFunction();
In this example, the function is hoisted to the top of the function scope.
This allows it to be called anywhere within the function before its actual declaration.
Difference Between var, let, and const Hoisting
The behavior of hoisting varies between var, let, and const.
Understanding these differences is crucial for writing predictable code.
Variables declared with var are hoisted and initialized with undefined.
This allows them to be accessed before their actual declaration.
console.log(varVar); // Outputs: undefined
var varVar = "Declared with var";
In this example, the var variable is hoisted and initialized with undefined.
Variables declared with let and const are hoisted but not initialized.
They exist in the Temporal Dead Zone until their actual declaration.
console.log(letVar); // ReferenceError: Cannot access 'letVar' before initialization
let letVar = "Declared with let";
console.log(constVar); // ReferenceError: Cannot access 'constVar' before initialization
const constVar = "Declared with const";
In this example, accessing let and const variables before their declaration results in a ReferenceError.
Hoisting and Strict Mode
Strict mode in JavaScript changes the behavior of hoisting in some cases.
Enabling strict mode can help catch common hoisting-related errors.
In strict mode, attempting to use a variable before its declaration will throw an error.
"use strict";
console.log(strictVar); // ReferenceError: 'strictVar' is not defined
var strictVar = "This is a strict mode variable.";
In this example, strict mode prevents the usage of a variable before its declaration.
Strict mode also affects the behavior of function declarations within blocks.
In non-strict mode, function declarations are hoisted to the top of their containing function or global scope.
In strict mode, they are scoped to the block in which they are declared.
Understanding strict mode can help you write more reliable and error-free code.
Function Expressions and Arrow Functions
Unlike function declarations, function expressions and arrow functions are not hoisted.
Attempting to use them before their declaration will result in an error.
console.log(expressionFunction()); // TypeError: expressionFunction is not a function
var expressionFunction = function() {
console.log("This is a function expression.");
};
console.log(arrowFunction()); // TypeError: arrowFunction is not a function
var arrowFunction = () => {
console.log("This is an arrow function.");
};
In this example, attempting to use function expressions and arrow functions before their declaration results in an error.
This behavior is crucial to understand to avoid unexpected errors in your code.
Common Mistakes and How to Avoid Them
Developers often make mistakes related to hoisting.
Understanding these common mistakes can help you avoid them in your code.
One common mistake is assuming that all variables and functions are hoisted.
In reality, only declarations are hoisted, not initializations or expressions.
Another common mistake is misunderstanding the scope within which hoisting occurs.
Variables and functions are hoisted to the top of their containing scope.
This can be the global scope, function scope, or block scope.
Finally, not following best practices like declaring variables at the top of their scope can lead to confusion.
Using let and const instead of var can help avoid unexpected behavior related to hoisting.
Examples of Debugging Hoisting Issues
Debugging hoisting issues can be challenging.
Here are some examples to help you understand how to debug such issues.
Suppose you encounter unexpected undefined values in your code.
This could be due to hoisting, where variables are accessed before their initialization.
console.log(debugVar); // Outputs: undefined
var debugVar = "This variable is hoisted.";
In this example, the variable is hoisted but initialized as undefined.
To debug this issue, ensure that you declare and initialize variables at the top of their scope.
Another example is encountering a ReferenceError with let and const variables.
This could be due to accessing variables within the Temporal Dead Zone.
console.log(debugLet); // ReferenceError: Cannot access 'debugLet' before initialization
let debugLet = "This is a let variable.";
In this example, the let variable is in the Temporal Dead Zone until its declaration.
To debug this issue, ensure that you declare let and const variables before accessing them.
Real-World Applications of Hoisting
Understanding hoisting can help you write more efficient and organized code.
Here are some real-world applications where hoisting is beneficial.
One application is in organizing your functions at the bottom of your script.
This improves the readability of your code by separating logic from function declarations.
initializeApp();
function initializeApp() {
console.log("App initialized.");
}
In this example, the function is declared at the bottom, but it can still be called at the top due to hoisting.
Another application is in using default parameter values in functions.
Hoisting ensures that functions can reference parameters declared later in the code.
function greet(name = getDefaultName()) {
console.log("Hello, " + name + "!");
}
function getDefaultName() {
return "Guest";
}
greet(); // Outputs: "Hello, Guest!"
In this example, the getDefaultName function is hoisted, allowing it to be used as a default parameter value.
Frequently Asked Questions
Does hoisting affect let and const variables?
Yes, but they are hoisted differently compared to var.
They are hoisted in the Temporal Dead Zone and cannot be accessed until their declaration.
Are arrow functions hoisted?
No, arrow functions assigned to variables are not hoisted.
Can hoisting lead to performance issues?
Hoisting itself does not lead to performance issues.
However, it can lead to code that is harder to read and debug.
Are class declarations hoisted?
No, class declarations are not hoisted.
Attempting to access a class before it is declared will result in a ReferenceError.
What errors can hoisting cause?
Hoisting can cause undefined values and ReferenceErrors if not understood properly.
It is important to be aware of how hoisting works to avoid these errors.
Following best practices and understanding the scope of hoisting can help you write more predictable code.
Shop more on Amazon